


How to Unmarshall Arbitrary JSON Data in Go with a Dynamic Code-Driven Schema?
Unmarshalling Arbitrary Data with JSON and Go
You have a specific requirement: unmarshalling JSON data in a way that allows you to process it in parts or sections. To achieve this, you're envisioning a structure where the first half of the data, or "code," indicates how to interpret the second half. Two potential structs for the second half are Range and User.
The Challenge
The existing json package in Go provides convenient functions for marshalling and unmarshalling defined structs, but it doesn't cater to your need for arbitrary data.
Decoding the Solution
The solution involves introducing a json.RawMessage field (Payload) in your Message struct. This field allows you to delay parsing the data until you know the code specified in the first half.
Here's an example implementation in Go:
<code class="go">type Message struct { Code int Payload json.RawMessage } type Range struct { Start int End int } type User struct { ID int Pass int } // Custom unmarshalling function func MyUnmarshall(m []byte) { var message Message json.Unmarshal(m, &message) var payload interface{} switch message.Code { case 3: payload = new(User) case 4: payload = new(Range) } json.Unmarshal(message.Payload, payload) }</code>
Usage
You would use this solution by first unmarshalling the "top half" using the json.Unmarshal function. Then, based on the code retrieved from the "top half," you would unmarshall the "bottom half" using the json.Unmarshal function again, this time providing the target json.RawMessage object.
Example
<code class="go">json := []byte(`{"Code": 4, "Payload": {"Start": 1, "End": 10}}`) MyUnmarshall(json) // This will decode the data into a Range object</code>
Considerations
This technique allows you to handle arbitrary data unmarshalling in a structured and flexible manner. However, it introduces a runtime dependency on knowing the code upfront and the existence of matching structs for different codes.
The above is the detailed content of How to Unmarshall Arbitrary JSON Data in Go with a Dynamic Code-Driven Schema?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


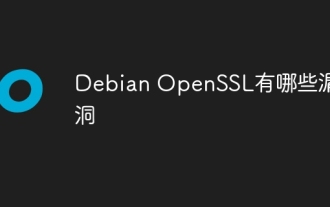
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
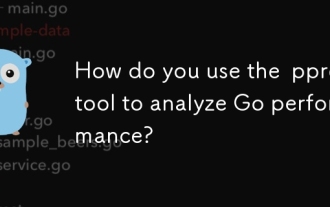
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
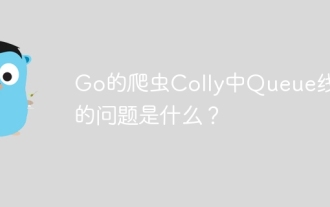
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
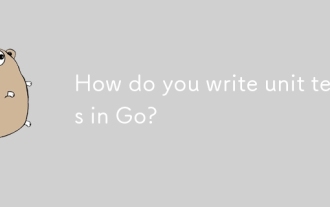
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
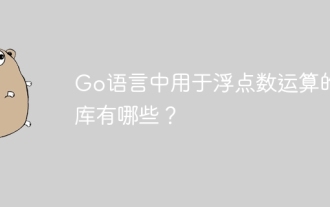
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
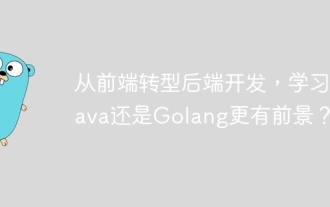
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
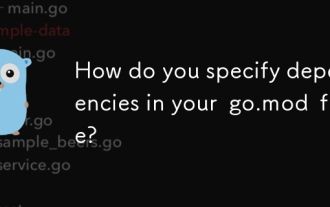
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
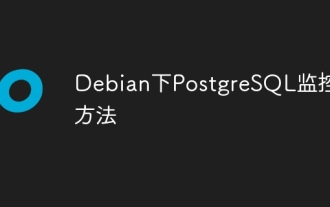
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
