


How to Efficiently Find Elements in a Vector of Structs Using std::find_if?
Nov 01, 2024 am 09:15 AMFinding Elements in a Vector of Structs using std::find
When working with complex data structures such as structs, searching through a vector of those elements can become challenging. In this context, the std::find function provides a solution for identifying specific elements within a vector.
Consider a struct definition like this:
<code class="cpp">struct monster { DWORD id; int x; int y; int distance; int HP; };</code>
Now, let's say we have a vector of monsters:
<code class="cpp">std::vector<monster> monsters;</code>
To search for an element based on a specific field within the struct, such as the monster's ID, we need to utilize std::find_if instead of std::find. std::find_if takes a predicate function as an argument, which allows us to define the search criteria.
Here's an example using the boost library:
<code class="cpp">it = std::find_if(bot.monsters.begin(), bot.monsters.end(), boost::bind(&monster::id, _1) == currentMonster);</code>
Or, if boost is not available, you can create your own find_id function object like this:
<code class="cpp">struct find_id : std::unary_function<monster, bool> { DWORD id; find_id(DWORD id) : id(id) {} bool operator()(monster const& m) const { return m.id == id; } }; it = std::find_if(bot.monsters.begin(), bot.monsters.end(), find_id(currentMonster));</code>
By using std::find_if and the appropriate predicate function, you can efficiently search through a vector of structs to find specific elements based on their member variables.
The above is the detailed content of How to Efficiently Find Elements in a Vector of Structs Using std::find_if?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
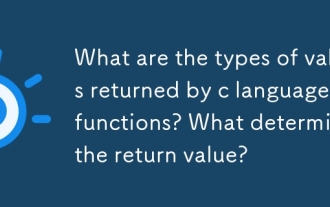
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
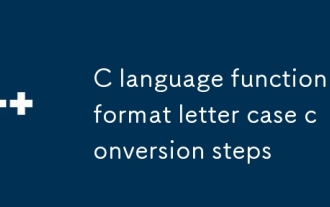
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
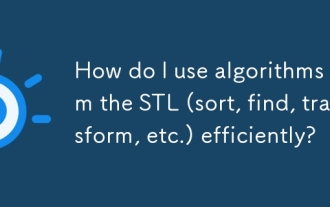
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
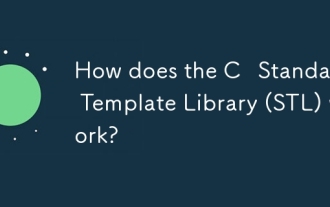
How does the C Standard Template Library (STL) work?
