How to Defer Evaluation of F-Strings in Python?
Nov 01, 2024 pm 03:43 PMDeferring Evaluation of F-Strings
In Python, f-strings offer a succinct way to generate formatted strings. However, sometimes it's useful to define templates elsewhere and import them as static strings. To efficiently evaluate these static strings as dynamic f-strings, we need a way to postpone their evaluation.
While the .format(**locals()) method can be used, it involves explicit variable expansion. To avoid this overhead, consider the following strategy:
<code class="python">def fstr(template): return eval(f'f&quot;&quot;&quot;{template}&quot;&quot;&quot;')</code>
This function takes a static template string and constructs an f-string dynamically at runtime. For instance, given the template:
<code class="python">template = "The current name is {name}"</code>
We can evaluate it using:
<code class="python">print(fstr(template)) # Output: The current name is foo</code>
Note that this method also supports expressions within curly braces, as exemplified by:
<code class="python">template = "The current name is {name.upper() * 2}" print(fstr(template)) # Output: The current name is FOOFOO</code>
By postponing the evaluation of f-strings using the fstr() function, developers can maintain code clarity and simplify template handling in Python.
The above is the detailed content of How to Defer Evaluation of F-Strings in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
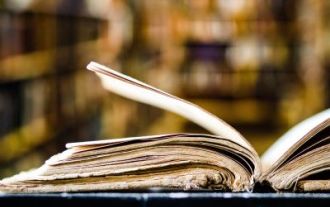
How to Use Python to Find the Zipf Distribution of a Text File
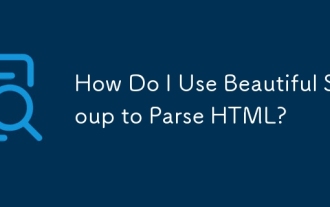
How Do I Use Beautiful Soup to Parse HTML?
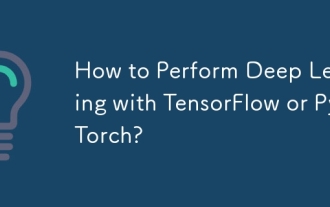
How to Perform Deep Learning with TensorFlow or PyTorch?
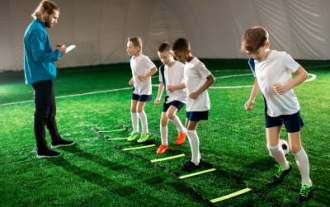
Introduction to Parallel and Concurrent Programming in Python
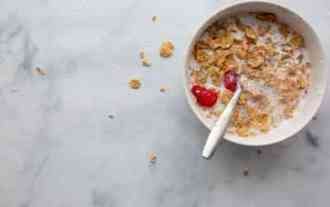
Serialization and Deserialization of Python Objects: Part 1
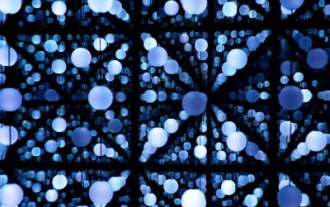
How to Implement Your Own Data Structure in Python
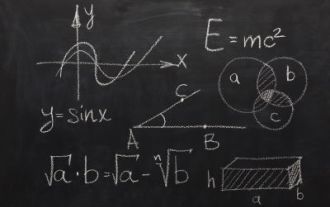
Mathematical Modules in Python: Statistics
