How to Determine the Method Set of an Interface in Go?
Determining the Method Set of an Interface in Go
When working with interfaces in Go, it can be useful to inspect the set of methods that an interface defines. This information can be invaluable for tasks such as validation, code generation, or simply understanding the intent of an interface.
Obtaining the Method Set Using Reflection
The Go language provides a powerful reflection package that allows you to examine the runtime representation of variables, including types. To retrieve the method set of an interface, we can use the following steps:
- Get the type information of the interface using reflect.TypeOf().
- Use the NumMethod() method on the type to get the number of methods defined by the interface.
- Iterate through the methods using the Method() method on the type, obtaining the method's name.
Here's a code snippet that demonstrates these steps:
<code class="go">package main import ( "fmt" "reflect" ) type Searcher interface { Search(query string) (found bool, err error) ListSearches() []string ClearSearches() (err error) } func main() { t := reflect.TypeOf(struct{ Searcher }{}) for i := 0; i < t.NumMethod(); i++ { fmt.Println(t.Method(i).Name) } }</code>
Running this program will output the names of the methods defined by the Searcher interface:
Search ListSearches ClearSearches
This technique allows you to determine the method set of an interface without knowing the concrete type that implements it.
The above is the detailed content of How to Determine the Method Set of an Interface in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










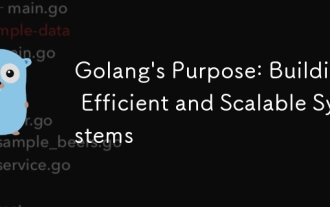
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
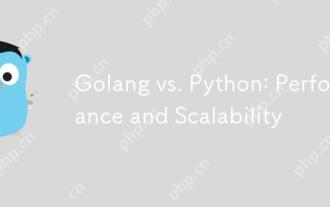
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
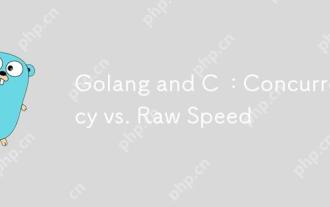
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
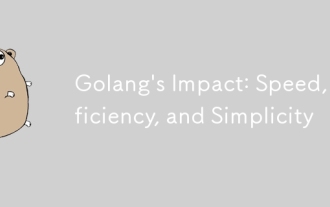
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
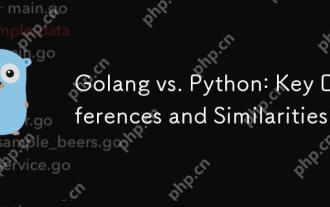
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
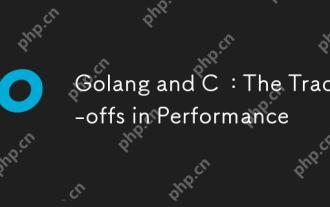
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
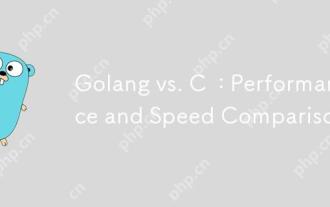
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
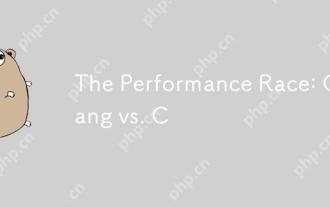
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
