


How to Count Elements with a Specific Class in jQuery and JavaScript?
Counting Elements by Class in jQuery: An Optimal Solution
Question:
How can we count the number of elements with the same class on a webpage using jQuery or JavaScript? This count is essential for dynamically assigning names to input forms based on the number of elements created.
jQuery Solution:
The most straightforward solution in jQuery is to utilize the .length property:
var numItems = $('.yourclass').length;
This code retrieves the number of elements with the class "yourclass" and assigns it to the numItems variable.
JavaScript Solution:
If you're using plain JavaScript, you can access the length property of the getElementsByClassName() method:
var numItems = document.getElementsByClassName('yourclass').length;
Optimization and Additional Tips:
It's prudent to check the length property before chaining further jQuery functions to ensure you have elements to work with. Consider the following:
var $items = $('.myclass'); if($items.length) { // Perform your jQuery operations on $items here }
Additionally, if you need to count elements within a specific sub-selection, you can use the .filter() method before checking the length property. For instance, to count odd elements:
var numOddItems = $('.myclass').filter(':odd').length;
The above is the detailed content of How to Count Elements with a Specific Class in jQuery and JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










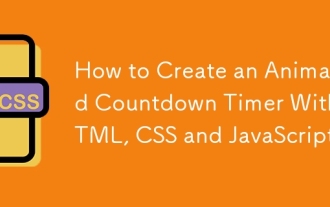
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
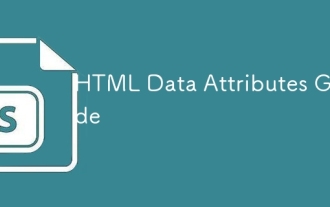
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
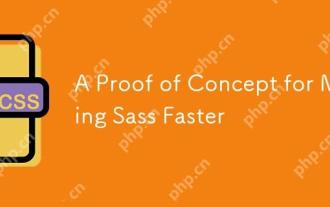
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
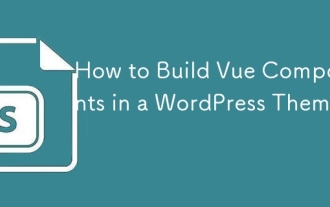
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
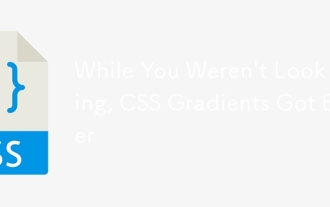
One thing that caught my eye on the list of features for Lea Verou's conic-gradient() polyfill was the last item:
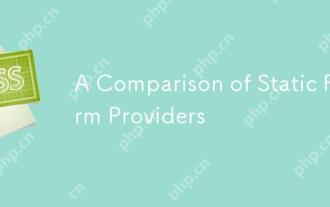
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
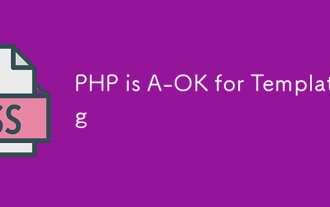
PHP templating often gets a bad rap for facilitating subpar code — but that doesn't have to be the case. Let’s look at how PHP projects can enforce a basic
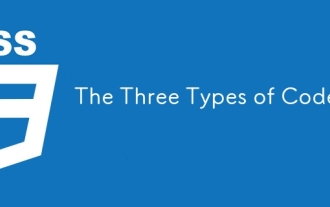
Every time I start a new project, I organize the code I’m looking at into three types, or categories if you like. And I think these types can be applied to
