


How do you append values to arrays within a map in Go while preserving object references?
Appending Values to Array within a Map in Go
When attempting to append values to arrays within a map, you may encounter difficulties setting references to Example objects.
In Go, the following code attempts to append values to an Example struct:
<code class="go">var MyMap map[string]Example type Example struct { Id []int Name []string } package main import ( "fmt" ) type Example struct { Id []int Name []string } func (data *Example) AppendExample(id int,name string) { data.Id = append(data.Id, id) data.Name = append(data.Name, name) } var MyMap map[string]Example func main() { MyMap = make(map[string]Example) MyMap["key1"] = Oferty.AppendExample(1,"SomeText") fmt.Println(MyMap) }</code>
However, this code is incorrect for several reasons:
- Initialization Error: Oferty is an undefined variable.
- Instance Creation: An instance of Example must be created before adding it to the map.
- Map Reference Issue: The map MyMap should contain references to Example instances, not copies.
The following corrected code addresses these issues:
<code class="go">package main import "fmt" type Example struct { Id []int Name []string } func (data *Example) AppendOffer(id int, name string) { data.Id = append(data.Id, id) data.Name = append(data.Name, name) } var MyMap map[string]*Example func main() { obj := &Example{[]int{}, []string{}} obj.AppendOffer(1, "SomeText") MyMap = make(map[string]*Example) MyMap["key1"] = obj fmt.Println(MyMap) }</code>
In this corrected code:
- An instance of Example (obj) is created before adding it to the map.
- The map MyMap contains references to Example instances using pointers (*Example).
- The method AppendOffer is used to append values to the array fields of the Example instance.
By implementing these corrections, the code correctly appends values to the arrays within the map while preserving object references.
The above is the detailed content of How do you append values to arrays within a map in Go while preserving object references?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










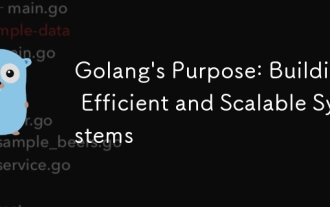
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
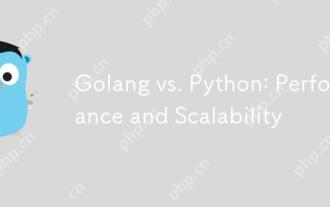
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
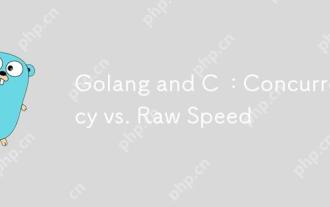
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
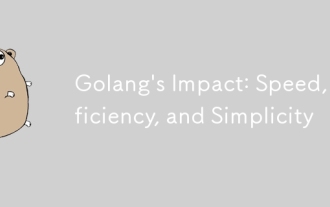
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
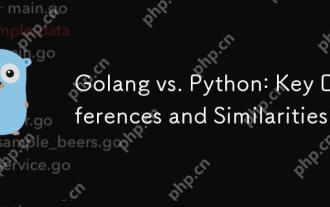
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
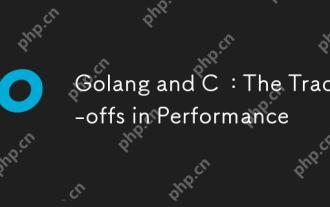
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
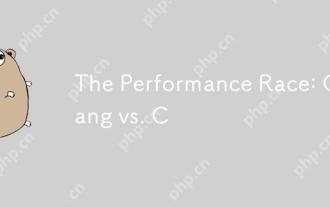
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
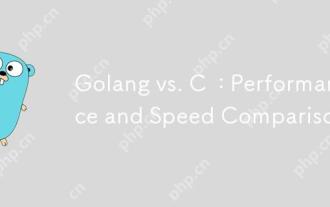
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
