How to Pass Parameters with Pandas read_sql?
Passing Parameters with Pandas read_sql
Overview
The Pandas read_sql function allows you to execute SQL queries and retrieve data from a database. One of its features is the ability to pass parameters to the query.
Parameter Syntax
Params can be passed as a list, tuple, or dict. The exact syntax depends on the database driver being used. Here are some common examples:
- ? or %s: Placeholder for a single value
- :1 or :name: Named placeholder for a single value
- %(name)s: Named placeholder using the string.format syntax
Using a Dictionary with Named Arguments
One option for passing parameters is to use a dictionary. This is supported by most drivers, including PostgreSQL with the psycopg2 driver. The key-value pairs in the dictionary correspond to the named placeholders in the query.
Example
To demonstrate the named argument approach, let's consider the following SQL query:
<code class="sql">select "Timestamp", "Value" from "MyTable" where "Timestamp" BETWEEN :dstart AND :dfinish</code>
Here's how you would pass parameters to this query using a dictionary:
<code class="python">params = {"dstart": datetime(2014, 6, 24, 16, 0), "dfinish": datetime(2014, 6, 24, 17, 0)} df = psql.read_sql( "select \"Timestamp\",\"Value\" from \"MyTable\" where \"Timestamp\" BETWEEN %(dstart)s AND %(dfinish)s", db, params=params, index_col=["Timestamp"], )</code>
In this example, the params dictionary provides values for the named placeholders :dstart and :dfinish. The %(name)s syntax ensures that the values are inserted correctly into the query.
The above is the detailed content of How to Pass Parameters with Pandas read_sql?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




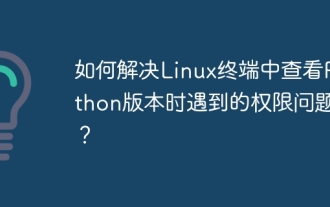
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
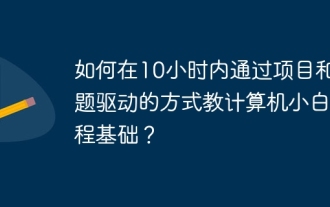
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
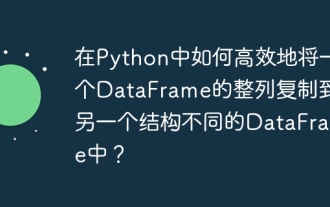
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
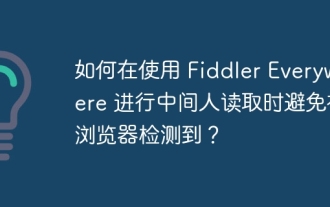
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
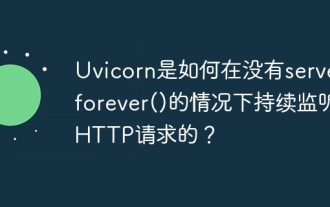
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
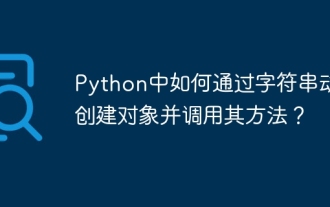
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
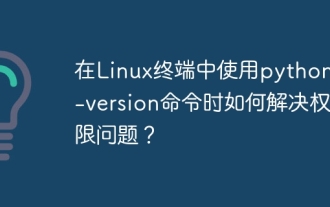
Using python in Linux terminal...
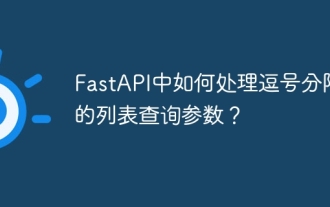
Fastapi ...
