How Can Interfaces Enhance Plugin Architecture in Go?
Plugin Architecture in Go: Using Interfaces for Seamless Extensibility
In Go, events and plugins can be seamlessly integrated into your core application using the concept of interfaces. While Node.js utilizes EventEmitter for extensibility, Go provides an alternative approach through the use of interfaces and a plugin registry.
Implementing Plugins
To define a plugin, create an interface that specifies the methods the plugin must implement. For instance, consider the following interfaces:
<code class="go">type DoerPlugin interface { DoSomething() } type FooerPlugin interface { Foo() }</code>
Plugin Registry
Establish a central repository for plugins in your core application, where plugins can be registered by type. Here's a simple implementation:
<code class="go">package plugin_registry var Fooers []FooerPlugin var Doers []DoerPlugin</code>
Provide methods to add plugins to the registry, allowing external modules to register themselves:
<code class="go">func RegisterFooer(f FooerPlugin) { Fooers = append(Fooers, f) } func RegisterDoer(d DoerPlugin) { Doers = append(Doers, d) }</code>
Integrating Plugins
By importing the plugin module into your main application, you automatically register the plugins defined within. Go's "init" function will register plugins at package initialization:
<code class="go">package main import ( "github.com/myframework/plugin_registry" _ "github.com/d00dzzzzz/myplugin" // Imports the plugin module for registration )</code>
Usage in Core Application
Within the core application, you can interact with plugins effortlessly:
<code class="go">func main() { for _, d := range plugin_registry.Doers { d.DoSomething() } for _, f := range plugin_registry.Fooers { f.Foo() } }</code>
Conclusion
This approach exemplifies how interfaces and a central registry can facilitate plugin integration in Go, providing a flexible and extensible architecture. While events can be incorporated into this framework, it demonstrates that interfaces offer a robust mechanism for plugin-based extensibility.
The above is the detailed content of How Can Interfaces Enhance Plugin Architecture in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










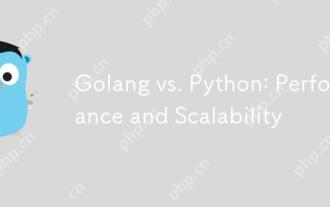
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
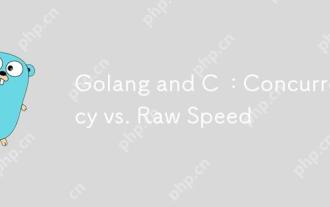
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
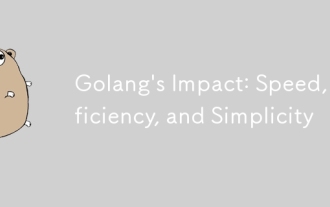
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
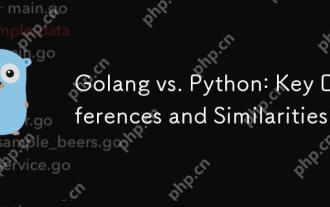
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
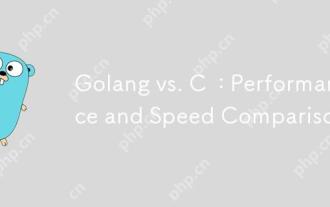
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
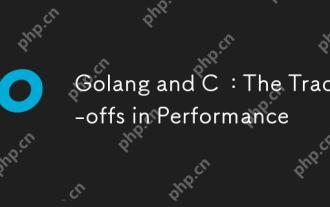
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
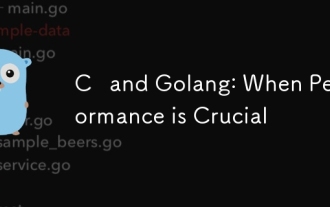
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
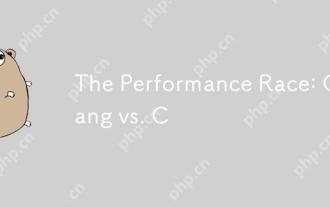
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
