Building ErgoVision: A Developers Journey in AI Safety
Introduction
Hey dev community! ? I'm excited to share the journey of building ErgoVision, an AI-powered system that's making workplaces safer through real-time posture analysis. Let's dive into the technical challenges and solutions!
The Challenge
When SIIR-Lab at Texas A&M University approached me about building a real-time posture analysis system, we faced several key challenges:
- Real-time processing requirements
- Accurate pose estimation
- Professional safety standards
- Scalable implementation
Technical Stack
# Core dependencies import mediapipe as mp import cv2 import numpy as np
Why This Stack?
- MediaPipe: Robust pose detection
- OpenCV: Efficient video processing
- NumPy: Fast mathematical computations
Key Implementation Challenges
1. Real-time Processing
The biggest challenge was achieving real-time analysis. Here's how we solved it:
def process_frame(self, frame): # Convert to RGB for MediaPipe rgb_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) results = self.pose.process(rgb_frame) if results.pose_landmarks: # Process landmarks self.analyze_pose(results.pose_landmarks) return results
2. Accurate Angle Calculation
def calculate_angle(self, a, b, c): vector1 = np.array([a[0] - b[0], a[1] - b[1], a[2] - b[2]]) vector2 = np.array([c[0] - b[0], c[1] - b[1], c[2] - b[2]]) # Handle edge cases if np.linalg.norm(vector1) == 0 or np.linalg.norm(vector2) == 0: return 0.0 cosine_angle = np.dot(vector1, vector2) / ( np.linalg.norm(vector1) * np.linalg.norm(vector2) ) return np.degrees(np.arccos(np.clip(cosine_angle, -1.0, 1.0)))
3. REBA Score Implementation
def calculate_reba_score(self, angles): # Initialize scores neck_score = self._get_neck_score(angles['neck']) trunk_score = self._get_trunk_score(angles['trunk']) legs_score = self._get_legs_score(angles['legs']) # Calculate final score return neck_score + trunk_score + legs_score
Lessons Learned
- Performance Optimization
- Use NumPy for vector calculations
- Implement efficient angle calculations
Optimize frame processing
Error Handling
def safe_angle_calculation(self, landmarks): try: angles = self.calculate_angles(landmarks) return angles except Exception as e: self.log_error(e) return self.default_angles
- Testing Strategy
- Unit tests for calculations
- Integration tests for video processing
- Performance benchmarking
Results
Our implementation achieved:
- 30 FPS processing
- 95% pose detection accuracy
- Real-time REBA scoring
- Comprehensive safety alerts
Code Repository Structure
ergovision/ ├── src/ │ ├── analyzer.py │ ├── pose_detector.py │ └── reba_calculator.py ├── tests/ │ └── test_analyzer.py └── README.md
Future Improvements
- Performance Enhancements
# Planned optimization @numba.jit(nopython=True) def optimized_angle_calculation(self, vectors): # Optimized computation pass
- Feature Additions
- Multi-camera support
- Cloud integration
- Mobile apps
Get Involved!
- Star our repository
- Try the implementation
- Contribute to development
- Share your feedback
Resources
- GitHub Repository
Happy coding! ?
The above is the detailed content of Building ErgoVision: A Developers Journey in AI Safety. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




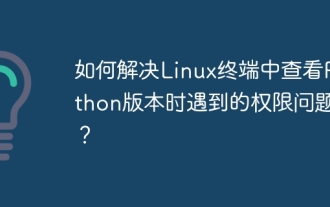
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
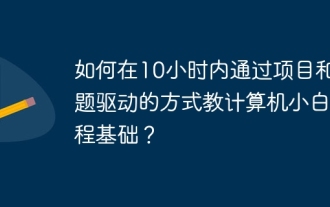
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
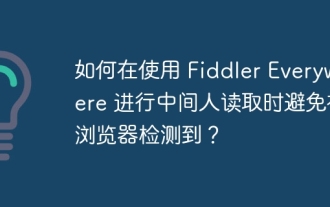
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
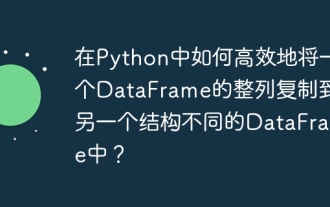
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
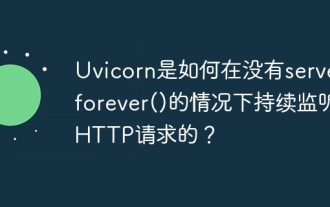
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
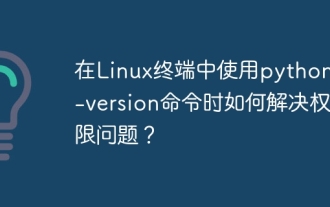
Using python in Linux terminal...
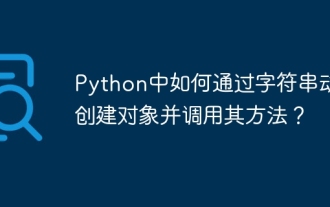
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
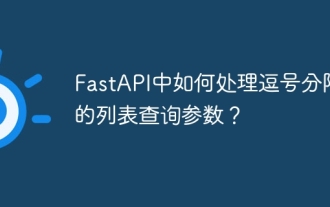
Fastapi ...
