Implicit vs. Explicit Waits in Selenium: When Should I Use Which?
How to Implement Delays in Selenium-WebDriver using Java: Implicit vs. Explicit Waits
In your Java Selenium-WebDriver project, you have encountered challenges with element location. You had added both implicit wait and a Thread.sleep, and while the latter resolved the issue, you seek a more suitable approach.
Implicit Wait vs. Explicit Wait
Selenium-WebDriver provides two types of waits:
- Implicit Wait: Configured once for the driver, it applies a waiting period before every WebDriver command.
- Explicit Wait: Used for specific conditions, it waits until a condition is met before proceeding.
Comparison
While implicit wait is convenient, it can lead to unnecessary delays if the element loads quickly. Explicit wait, on the other hand, provides more control and flexibility.
Recommended Approach: Explicit Wait
In your scenario, explicit wait is a more suitable solution due to the varying load times of your application's User Interface. The code example provided below demonstrates the use of an explicit wait:
<code class="java">import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; public class ExplicitWaitExample { public static void main(String[] args) { WebDriver driver = getDriver(); WebElement textbox = new WebDriverWait(driver, 30) .until(ExpectedConditions.presenceOfElementLocated(By.id("textbox"))); } }</code>
This approach ensures that Selenium waits until the textbox element is present on the page before continuing, allowing you to avoid the use of a fixed waiting period.
The above is the detailed content of Implicit vs. Explicit Waits in Selenium: When Should I Use Which?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
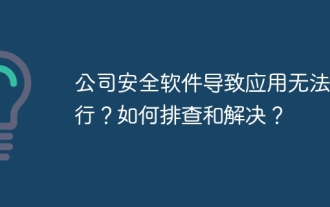
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
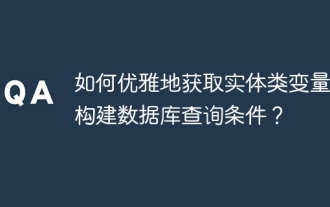
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
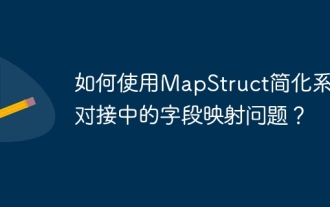
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
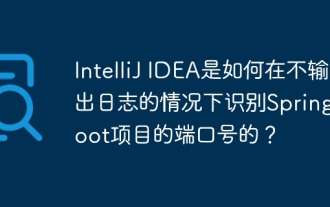
Start Spring using IntelliJIDEAUltimate version...
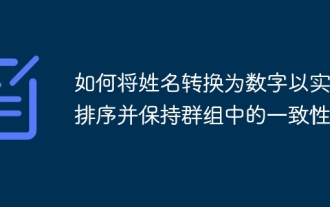
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
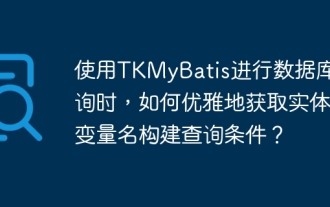
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
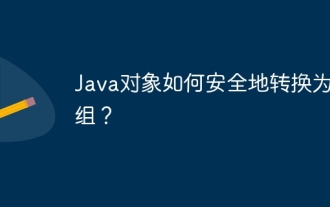
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
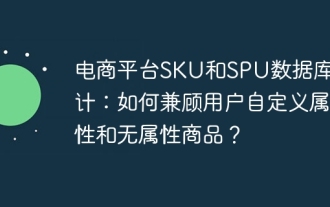
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
