


How do you check for element presence in slices of different types in Go?
How to Generically Check for Element Presence in a Slice in Go
In Go, determining whether a slice contains a specific element can be a common scenario. However, there is no built-in method to perform this generic check across different slice types.
Failed Attempt with Interface{}
An attempt to use the interface{} type as a generic solution, as shown below, may seem plausible:
<code class="go">func sliceContains(slice []interface{}, elem interface{}) bool { for _, item := range slice { if item == elem { return true } } return false }</code>
However, comparing values of different types (interface{}) can lead to incorrect results.
Generic Solution with Reflection
To achieve a truly generic solution, reflection can be employed. The following function uses reflection to iterate over the slice and compare each element to the target element:
<code class="go">func Contains(slice, elem interface{}) bool { sv := reflect.ValueOf(slice) // Check that slice is actually a slice/array. if sv.Kind() != reflect.Slice && sv.Kind() != reflect.Array { return false } // Iterate the slice for i := 0; i < sv.Len(); i++ { // Compare elem to the current slice element if elem == sv.Index(i).Interface() { return true } } // Nothing found return false }</code>
This solution allows you to perform generic element checks on slices of any type.
Performance Considerations
While the generic Contains function provides the desired functionality, it comes at a significant performance cost. Benchmarking against a non-generic equivalent function yields a slowdown factor of approximately 50x. Therefore, it is crucial to evaluate the performance implications before using reflection for generic element checks.
The above is the detailed content of How do you check for element presence in slices of different types in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










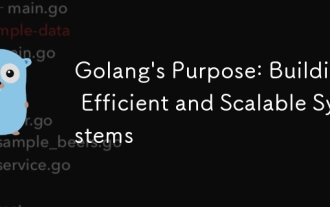
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
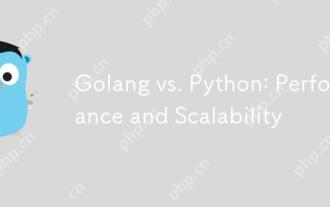
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
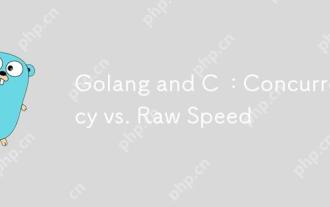
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
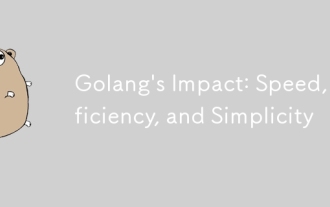
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
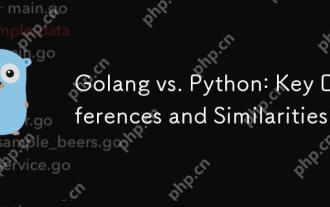
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
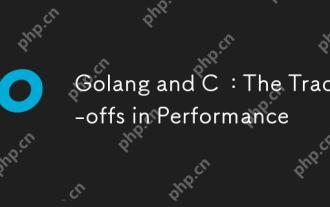
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
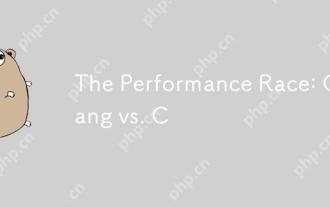
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
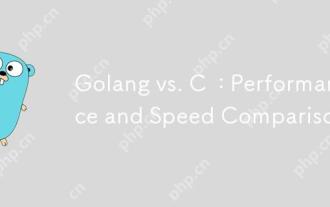
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
