


How to Efficiently Check for Multiple Values in a List in Python: All vs Sets?
Testing Multiple Values for Membership in a List
When attempting to check if multiple values belong to a list, you may encounter unexpected results. For instance, testing membership using Python's ',' operator may return an unexpected tuple.
<code class="python">'a','b' in ['b', 'a', 'foo', 'bar'] ('a', True)</code>
Python's "all" Function
To accurately test membership of multiple values, utilize Python's all function in conjunction with list comprehension, as demonstrated below:
<code class="python">all(x in ['b', 'a', 'foo', 'bar'] for x in ['a', 'b']) True</code>
Alternative Approaches
Sets
Sets can also be employed for membership testing. However, they have limitations. For example, they cannot handle unhashable elements like lists.
<code class="python">{'a', 'b'} <= {'a', 'b', 'foo', 'bar'} True {'a', ['b']} <= {'a', ['b'], 'foo', 'bar'} Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: unhashable type: 'list'</code>
Speed Comparisons
In most cases, subset testing using the all function is faster than using sets. However, this advantage diminishes when the list is large and contains many non-hashable elements. Moreover, if the test items are already stored in a set, using the subset test will be significantly faster.
Conclusion
When testing membership of multiple values in a list, the all function with list comprehension is the recommended approach. Sets can be useful in certain situations, but their limitations should be considered. The most optimal approach depends on the specific context and data being tested.
The above is the detailed content of How to Efficiently Check for Multiple Values in a List in Python: All vs Sets?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










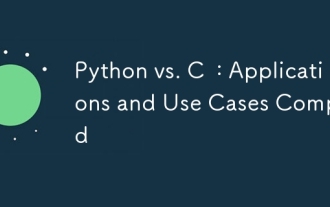
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
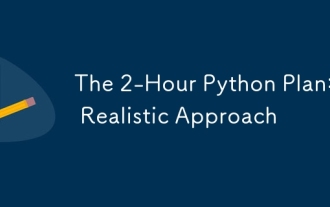
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
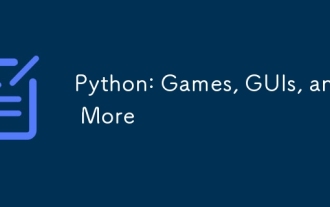
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
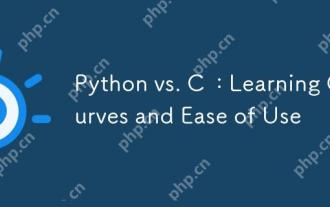
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
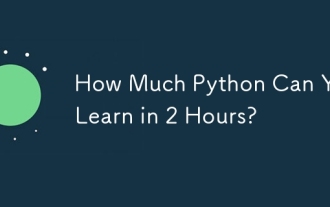
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
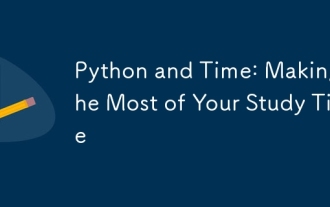
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
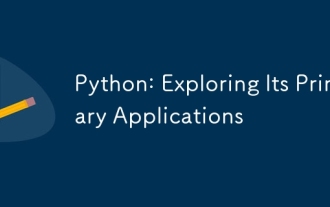
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
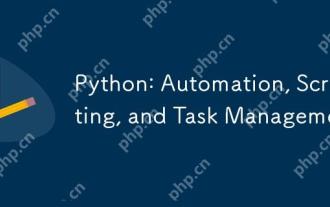
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
