


How Can Go\'s Concurrency Principles Be Applied to Create Safe and Efficient Shared Maps?
Efficient Shared Map Implementation Techniques in Go
Concurrent access to shared data structures requires careful consideration to ensure data integrity. Consider the case of a map that is simultaneously accessed by multiple goroutines, as seen in the example below.
<code class="go">func getKey(r *http.Request) string { ... } values := make(map[string]int) http.HandleFunc("/get", func(w http.ResponseWriter, r *http.Request) { key := getKey(r) fmt.Fprint(w, values[key]) }) http.HandleFunc("/set", func(w http.ResponseWriter, r *http.Request) { key := getKey(r) values[key] = rand.Int() })</code>
Direct manipulation of the map through concurrent writes can lead to data inconsistency. Employing a mutex, as demonstrated below, addresses the atomicity issue but introduces another problem.
<code class="go">func getKey(r *http.Request) string { ... } values := make(map[string]int) var lock sync.RWMutex http.HandleFunc("/get", func(w http.ResponseWriter, r *http.Request) { key := getKey(r) lock.RLock() fmt.Fprint(w, values[key]) lock.RUnlock() }) http.HandleFunc("/set", func(w http.ResponseWriter, r *http.Request) { key := getKey(r) lock.Lock() values[key] = rand.Int() lock.Unlock() })</code>
While mutexes provide reliable synchronization, they introduce the complexity of manual locking and unlocking. A more idiomatic approach in Go involves utilizing channels. By default, it is recommended to prioritize channels over mutexes, as exemplified by Go's motto: "Share memory by communicating, don't communicate by sharing memory."
Here are some key considerations:
- Use channels whenever possible to simplify synchronization and eliminate the need for explicit locking.
- When necessary, consider using reference counting through a mutex, but default to using channels for concurrency control.
- Consult Rob Pike's article for a comprehensive guide to building safe maps for concurrent usage.
- Remember Go's philosophy: "Concurrency Simplifies Synchronization" and "Only one goroutine has access to the value at any given time."
The above is the detailed content of How Can Go\'s Concurrency Principles Be Applied to Create Safe and Efficient Shared Maps?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


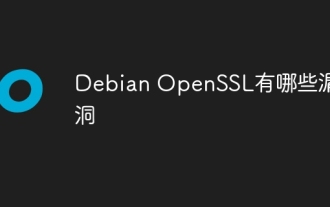
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
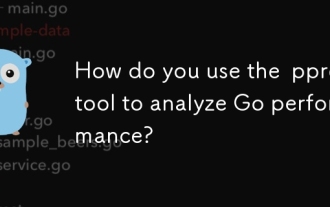
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
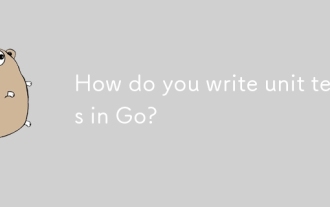
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
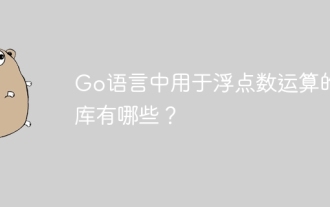
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
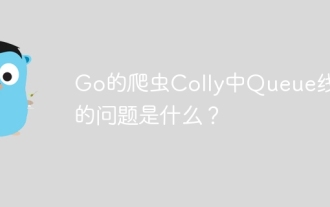
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
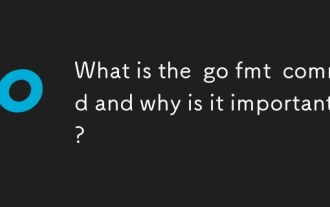
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
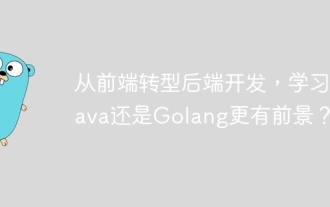
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
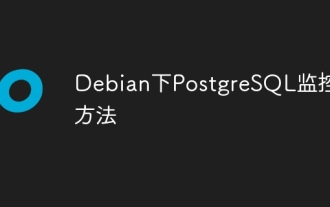
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
