How to Return Custom Objects from Spring Data JPA GROUP BY Queries?
Custom Object Return in Spring Data JPA GROUP BY Queries
Introduction
Retrieving data as custom objects from Spring Data JPA GROUP BY queries enhances data presentation and simplifies further processing. This guide explores how to achieve this, showcasing solutions for both JPQL and native queries.
JPQL Queries
JPQL queries within the JPA specification offer native support for returning custom objects.
Step 1: Create a Custom Bean
Define a simple bean class to represent the desired output structure:
1 2 3 4 5 6 |
|
Step 2: Return Bean Instances
Update the repository method to return instances of the custom bean:
1 2 3 4 |
|
Native Queries
While native queries lack direct support for the new keyword, Spring Data Projection interfaces provide an alternative solution:
Step 1: Define a Projection Interface
Create a projection interface with properties corresponding to the desired output:
1 2 3 4 |
|
Step 2: Return Projected Properties
Update the repository method to return projected properties:
1 2 3 4 5 |
|
Employ the SQL AS keyword to map result fields to projection properties seamlessly.
The above is the detailed content of How to Return Custom Objects from Spring Data JPA GROUP BY Queries?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










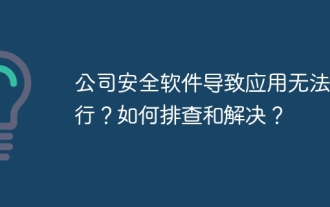
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
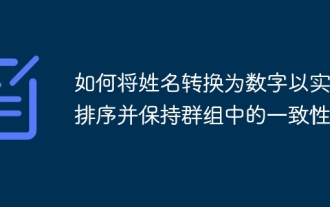
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
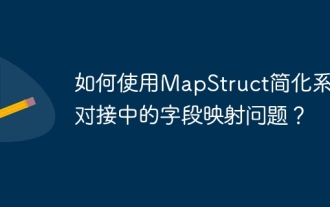
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
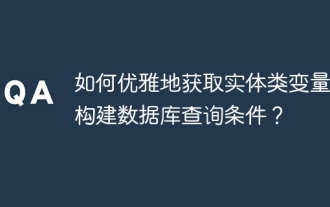
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
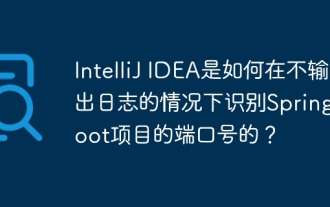
Start Spring using IntelliJIDEAUltimate version...
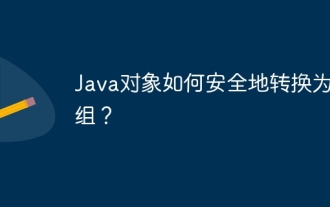
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
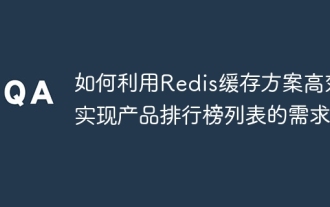
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
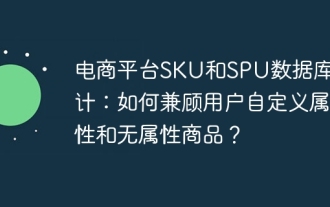
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
