


The following are several English question and answer titles that match the content of the article: * Why Am I Unable to Retrieve Data from a MySQL Database Through SSH.NET? * Troubleshooting MySQL Connection Issues with SSH.NET: A Case Study * How to Correctly Connect to a Rem
Connection to MySQL from .NET using SSH.NET Library
Issue:
An ASP.NET/C# web page is attempting to query a MySQL database on a remote server over an SSH connection using the Renci.SshNet and mysql-connector-net libraries. However, the code is not retrieving any data from the database.
Code Snippet:
The C# code provided attempts to establish an SSH connection, forward a port, and then connect to the MySQL database. However, it fails to retrieve any data from the database.
<code class="csharp">using(var client = new SshClient("ssh server id", "sshuser", "sshpassword")) // establishing ssh connection to server where MySql is hosted { client.Connect(); if (client.IsConnected) { var portForwarded = new ForwardedPortLocal("127.0.0.1", 3306, "127.0.0.1", 3306); client.AddForwardedPort(portForwarded); portForwarded.Start(); using (MySqlConnection con = new MySqlConnection("SERVER=127.0.0.1;PORT=3306;UID=someuser;PASSWORD=somepass;DATABASE=Dbname")) { using (MySqlCommand com = new MySqlCommand("SELECT * FROM cities", con)) { com.CommandType = CommandType.CommandText; DataSet ds = new DataSet(); MySqlDataAdapter da = new MySqlDataAdapter(com); da.Fill(ds); foreach (DataRow drow in ds.Tables[0].Rows) { Console.WriteLine("From MySql: " + drow[1].ToString()); } } } client.Disconnect(); } else { Console.WriteLine("Client cannot be reached..."); } }</code>
Solution:
To fix the issue, the following modifications can be made to the code:
- The connection string used to connect to the MySQL database has an incorrect syntax. Change the connection string to the following:
MySqlConnectionStringBuilder connBuilder = new MySqlConnectionStringBuilder(); connBuilder.AllowBatch = true; connBuilder.Server = "portal.RemoteServer.edu"; connBuilder.Port = 3306; connBuilder.UserID = "RemoteServerUsername"; connBuilder.Password = "RemoteServerPassword"; connBuilder.Database = "DatabaseName";
- The SSH connection is not established properly. Ensure that the SSH connection parameters are correct and that the client is successfully connecting to the remote server.
Modified Code:
<code class="csharp">using(var client = new SshClient("portal.RemoteServer.edu", "RemoteServerUsername", "RemoteServerPassword")) // establishing ssh connection to server where MySql is hosted { client.Connect(); if (client.IsConnected) { var portForwarded = new ForwardedPortLocal("127.0.0.1", 3306, "127.0.0.1", 3306); client.AddForwardedPort(portForwarded); portForwarded.Start(); using (MySqlConnection con = new MySqlConnection(connBuilder.ConnectionString)) { using (MySqlCommand com = new MySqlCommand("SELECT * FROM Clients", con)) { com.CommandType = CommandType.CommandText; DataSet ds = new DataSet(); MySqlDataAdapter da = new MySqlDataAdapter(com); da.Fill(ds); foreach (DataRow drow in ds.Tables[0].Rows) { Console.WriteLine("From MySql: " + drow[1].ToString()); } } } client.Disconnect(); } else { Console.WriteLine("Client cannot be reached..."); } }</code>
The above is the detailed content of The following are several English question and answer titles that match the content of the article: * Why Am I Unable to Retrieve Data from a MySQL Database Through SSH.NET? * Troubleshooting MySQL Connection Issues with SSH.NET: A Case Study * How to Correctly Connect to a Rem. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
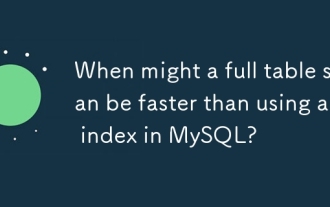
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
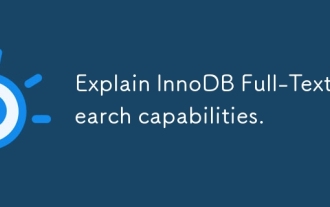
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
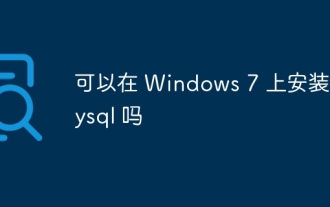
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
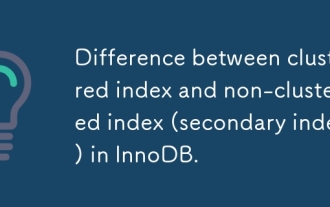
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
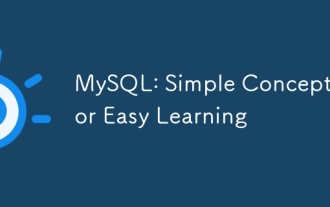
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
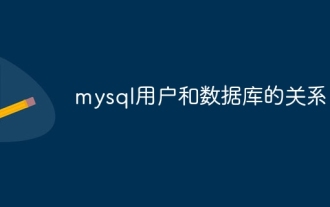
In MySQL database, the relationship between the user and the database is defined by permissions and tables. The user has a username and password to access the database. Permissions are granted through the GRANT command, while the table is created by the CREATE TABLE command. To establish a relationship between a user and a database, you need to create a database, create a user, and then grant permissions.
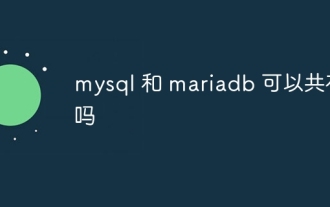
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
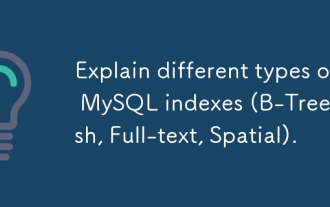
MySQL supports four index types: B-Tree, Hash, Full-text, and Spatial. 1.B-Tree index is suitable for equal value search, range query and sorting. 2. Hash index is suitable for equal value searches, but does not support range query and sorting. 3. Full-text index is used for full-text search and is suitable for processing large amounts of text data. 4. Spatial index is used for geospatial data query and is suitable for GIS applications.
