


Why am I getting \'panic: runtime error: index out of range\' even though my Go slice has a non-zero capacity?
Troubleshooting "panic: runtime error: index out of range" When Array Length Is Not Null
In Go, when working with arrays or slices, it's crucial to understand the concept of length and capacity. In the given code snippet, the issue stems from initializing the result array using make([]string, 0, 4).
Understanding the Role of make([]string, 0, 4)
make([]string, 0, 4) creates a slice of type []string with an initial length of 0 and a capacity of 4. Capacity defines the maximum number of elements that can be stored in the slice without reallocating memory.
Distinguishing Between Length and Capacity
Length indicates the number of elements currently present in the slice, while capacity represents the maximum size it can hold. If the slice already holds enough elements, adding more items within the capacity will not cause a reallocation. However, exceeding the capacity will trigger a reallocation, which involves creating a new underlying array and copying the existing elements.
In your code, make([]string, 0, 4) initializes an empty slice with a capacity of 4. Attempting to access elements beyond the current length (index 0) will result in a runtime error because there are no elements at that index.
Resolving the Issue
The error can be resolved by either initializing the slice with a sufficient initial length or using append to dynamically grow the slice as needed.
Option 1: Initialize with a Sufficient Length
If you know the approximate number of elements that will be stored in the slice, you can initialize it with an appropriate length. For example, result := make([]string, 4) initializes a slice with a length and capacity of 4.
Option 2: Use Append
Append appends elements to the end of a slice. If the slice has sufficient capacity, it is resliced to accommodate the new elements. If not, a new underlying array is allocated. By using append repeatedly, you can gradually grow the slice as needed without worrying about exceeding the capacity.
Simplified Code Using Append
Here's a simplified version of your code using append:
<code class="go">package main import ( "fmt" "strings" ) func main() { strs := strings.Fields("Political srt") var result []string for _, s := range strs { if strings.ContainsAny(s, "eaiu") { result = append(result, s) } } fmt.Println(result) }</code>
The above is the detailed content of Why am I getting \'panic: runtime error: index out of range\' even though my Go slice has a non-zero capacity?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










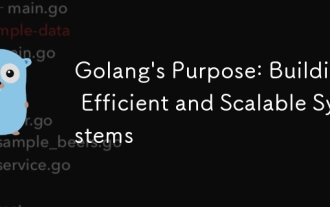
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
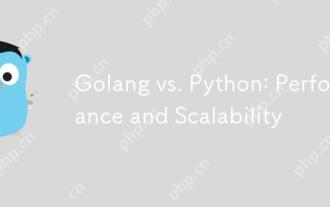
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
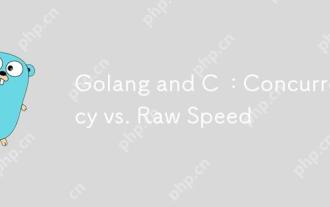
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
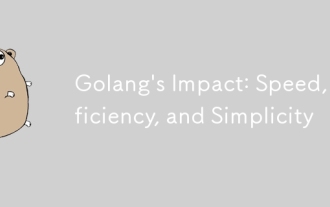
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
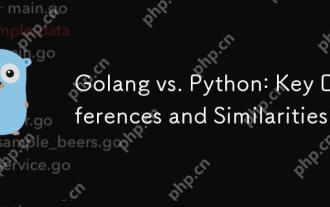
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
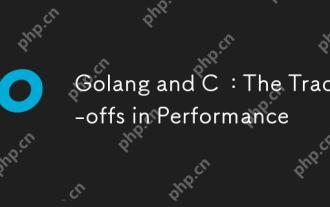
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
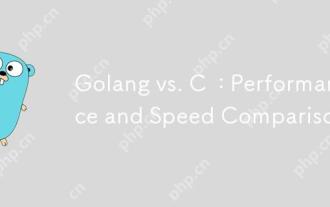
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
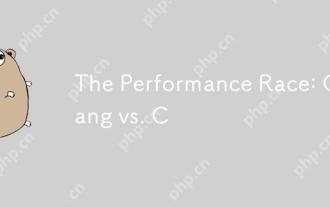
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
