How to Capture Screenshots in Linux Using Python?
How to Capture Screenshots Using Python on Linux
When you need to unobtrusively capture screenshots for automation or documentation purposes, using Python on a Linux system is an effective solution. Here's how you can do it:
Understanding the Task
The goal is to create a Python script capable of taking screenshots and saving them without any visible user interface (GUI) intervention.
Leveraging GTK for Screenshotting
To achieve this, we'll harness the power of GTK (GIMP Toolkit), a cross-platform graphics library commonly used in Linux environments. GTK provides access to the underlying graphics stack, enabling us to perform tasks such as screenshot capture.
Diving into the Python Code
Here's a concise and robust Python script that utilizes GTK for screenshotting:
<code class="python">import gtk.gdk # Get the default root window w = gtk.gdk.get_default_root_window() # Determine the screen size sz = w.get_size() # Create a pixbuf object pb = gtk.gdk.Pixbuf(gtk.gdk.COLORSPACE_RGB, False, 8, sz[0], sz[1]) # Capture the window screenshot pb = pb.get_from_drawable(w, w.get_colormap(), 0, 0, 0, 0, sz[0], sz[1]) if pb: # Save the screenshot as a PNG file pb.save("screenshot.png", "png") print("Screenshot saved to screenshot.png.") else: print("Unable to get the screenshot.")</code>
Key Points
- No External Dependencies: This script doesn't require additional tools like scrot or ImageMagick, making it a standalone solution.
- Cross-Platform Compatibility: GTK ensures that the script works across various X-based Linux environments.
- Unobtrusive Operation: The screenshot is captured seamlessly without any visible GUI interactions.
The above is the detailed content of How to Capture Screenshots in Linux Using Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










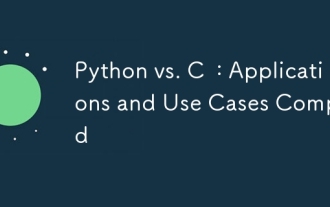
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
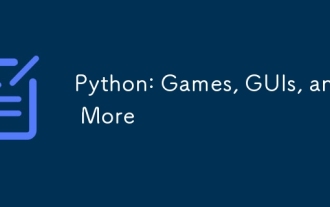
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
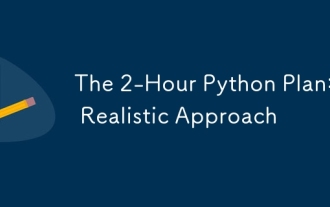
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
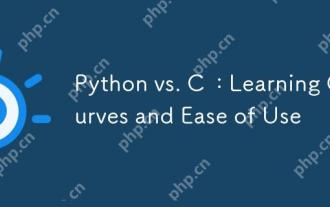
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
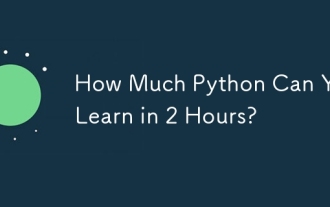
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
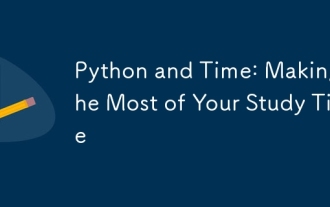
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
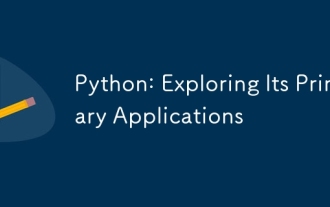
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
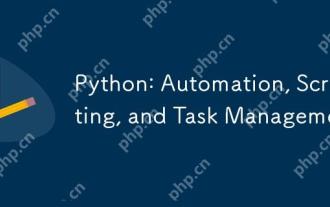
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
