How to Efficiently Count Character Frequencies in a String Using Java?
Nov 02, 2024 pm 12:34 PMCharacter Frequency in a String
Determining the frequency of each character in a string is a common programming task. In this context, a solution involving Java Map and Multiset approaches is outlined.
Java Map Approach
To create a map with each character as a key and its count as a value, the following steps can be employed:
- Initialize an empty HashMap object.
- Iterate through the string character by character.
-
Check if the current character is already a key in the map.
- If yes, increment the existing value by 1.
- If no, add the character as a key with a value of 1.
Example:
<code class="java">Map<Character, Integer> map = new HashMap<>(); String s = "aasjjikkk"; for (int i = 0; i < s.length(); i++) { char c = s.charAt(i); Integer val = map.get(c); if (val != null) { map.put(c, val + 1); } else { map.put(c, 1); } }
Multiset Approach
Alternatively, the Guava Multiset implementation can be utilized to count character occurrences efficiently. Multisets allow for storing multiple instances of an element and automatically track their counts.
Example:
<code class="java">Multiset<Character> multiset = HashMultiset.create(); String s = "aasjjikkk"; for (char c : s.toCharArray()) { multiset.add(c); }</code>
The multiset object will contain the character counts as its values.
Both approaches effectively allow you to determine the frequency of each character in a string, offering flexibility and performance optimizations depending on your specific requirements.
The above is the detailed content of How to Efficiently Count Character Frequencies in a String Using Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
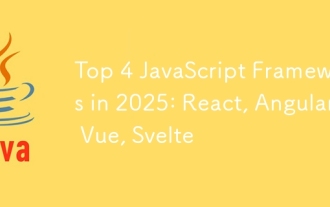
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
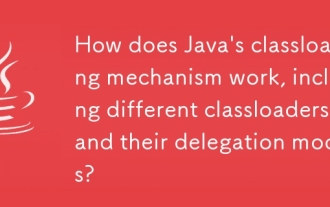
How does Java's classloading mechanism work, including different classloaders and their delegation models?
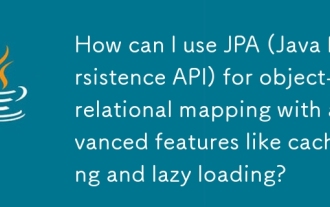
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
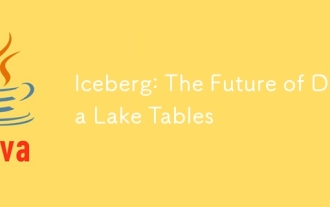
Iceberg: The Future of Data Lake Tables
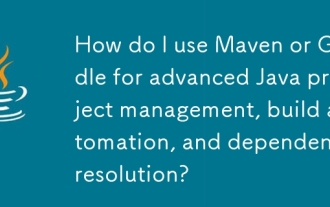
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
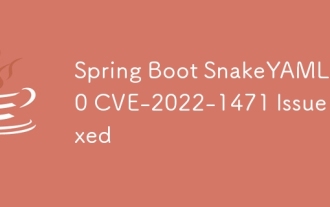
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
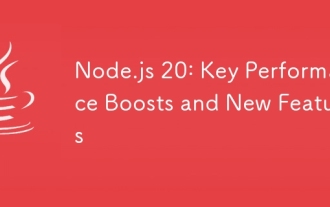
Node.js 20: Key Performance Boosts and New Features
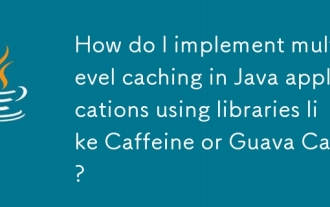
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
