How to Access ViewPager Fragment Methods from Activity?
Nov 02, 2024 pm 06:05 PMAccessing ViewPager Fragment Methods from Activity
ViewPager is widely used in Android applications to facilitate the management of multiple fragments within a single activity. However, accessing fragment methods directly from the host activity can be challenging. This article explores two approaches to achieve this, each with its own trade-offs.
First Approach: Using setUserVisibleHint
This approach triggers the sendGetRequest() method when a fragment becomes visible. However, it may introduce a noticeable lag in the swiping animation due to the timing of the method execution.
Second Approach: Using OnPageChangeListener
Using ViewPager's OnPageChangeListener offers a more precise and timely way to access fragment methods during page transitions. By adding a listener to the ViewPager within the host activity, you can target specific fragment pages and execute methods like sendGetRequest() when the page is selected.
Implementation
To effectively implement the second approach, you need to:
- Create a ViewPager.OnPageChangeListener and override its methods.
- Register the listener with the ViewPager using addOnPageChangeListener().
- Within the listener's onPageSelected() method, identify the current fragment and cast it to the appropriate class if necessary.
- Finally, invoke the desired method, such as sendGetRequest().
Example
ViewPager.OnPageChangeListener listener = new ViewPager.OnPageChangeListener() { public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) { } public void onPageSelected(int position) { Fragment fragment = mainPagerAdapter.fragments[position]; if (fragment != null ) { if (fragment instanceof MyFragment) { ((MyFragment) fragment).sendGetRequest(); } } } }; viewPager.addOnPageChangeListener(listener);
Note: It's important to cast the Fragment object to the correct class if it's not an instance of the general Fragment class. This is necessary to access class-specific methods.
Conclusion
Leveraging the OnPageChangeListener provides a robust way to control and interact with ViewPager fragments from the host activity, enabling you to send requests, modify data, or perform other operations in response to fragment page transitions.
The above is the detailed content of How to Access ViewPager Fragment Methods from Activity?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
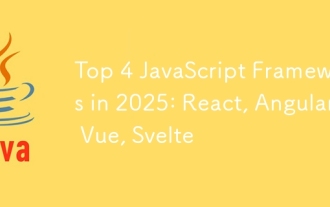
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
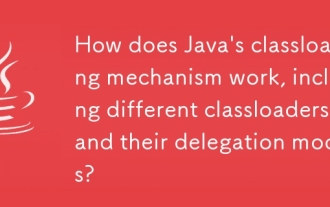
How does Java's classloading mechanism work, including different classloaders and their delegation models?
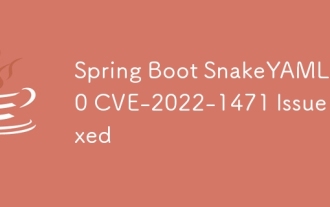
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
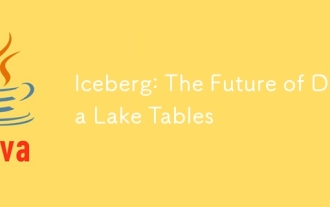
Iceberg: The Future of Data Lake Tables
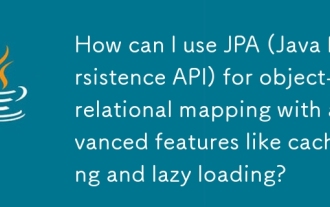
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
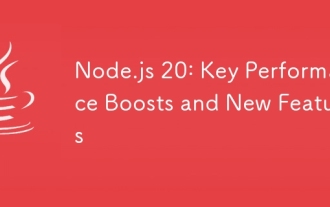
Node.js 20: Key Performance Boosts and New Features
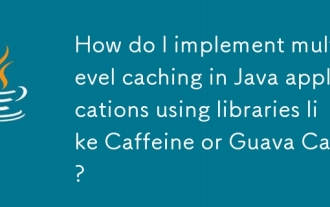
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
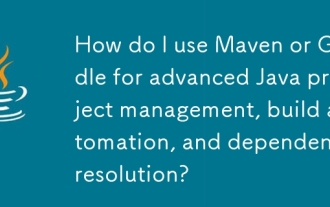
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
