How to Split Large Strings into N-Sized Chunks using JavaScript?
Splitting Large Strings into N-Sized Chunks in JavaScript
To efficiently split a large string into smaller chunks of size N, you can utilize the String.prototype.match method in JavaScript. This method enables you to apply a regular expression pattern to the string and extract matching substrings.
Using String.prototype.match:
To split a string into N-sized chunks, you can use the following regular expression pattern:
/.{1,n}/g
Where n represents the desired chunk size. For instance, to split a string into chunks of size 2, the pattern would be:
/.{1,2}/g
Example:
Consider the string "1234567890" and a chunk size of 2. Using the aforementioned pattern, you can split the string as follows:
"1234567890".match(/.{1,2}/g)
This will result in the following array:
["12", "34", "56", "78", "90"]
Handling Odd-Sized Chunks:
If the string size is not an exact multiple of the chunk size, the last chunk may be smaller than the desired size. For example, with a string of size 9 and a chunk size of 2, the resulting array would be:
["12", "34", "56", "78", "9"]
Reusable Function:
You can package the splitting logic into a reusable function:
function chunkString(str, length) { return str.match(new RegExp('.{1,' + length + '}', 'g')); }
Performance Considerations:
Although the match method is efficient in most cases, its performance may vary depending on the input string's size and the specific regular expression pattern used. It's worth noting that with large strings, splitting operations can take some time, so it's advisable to test and optimize your code accordingly.
The above is the detailed content of How to Split Large Strings into N-Sized Chunks using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


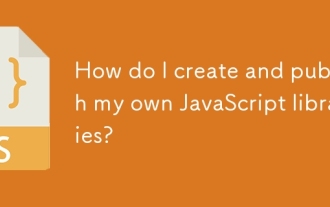
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
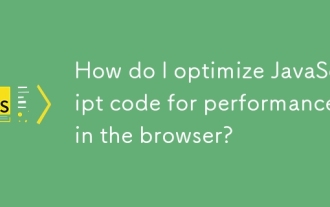
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
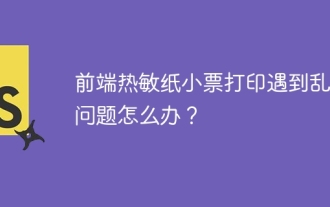
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
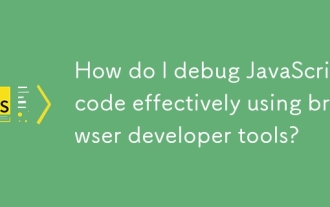
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
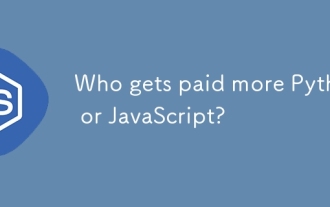
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
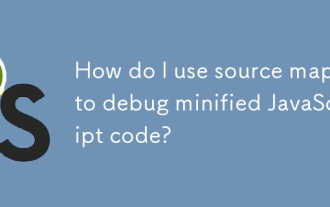
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
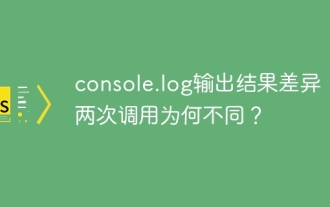
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
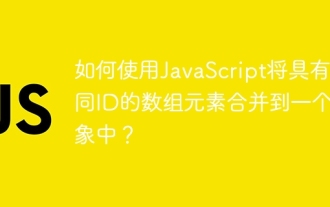
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
