How to Handle Negative Numbers in Modulo Operations in C/C /Obj-C?
Handling Negative Numbers in Modulo Calculations in C/C /Obj-C
In C-based languages, modulo operations using the "%" operator can exhibit unexpected behaviors when dealing with negative numbers. Specifically, the result can be different from that of mathematical modulo operations.
Problem Explanation
As a mathematical concept, modulo division returns the remainder when one number is divided by another. However, in C/C /Obj-C, the modulo operator prioritizes preserving the sign of the dividend (the first operand) over ensuring a positive remainder. This can lead to negative results even when the mathematical division would result in a positive remainder.
For example, consider the expression "(-1) % 8". Mathematically, this should return 7 because 8 goes into -1 once with a remainder of 7. However, in C/C , the result would be -1 because it maintains the sign of the dividend.
Solution with Implementation Considerations
To rectify this situation, a revised version of the modulo operator can be implemented to account for negative numbers:
<code class="c++">int customMod(int a, int b) { if (b < 0) { return -customMod(-a, -b); } int ret = a % b; if (ret < 0) { ret += b; } return ret; }</code>
In this optimized version, if one of the operands is negative, it reverses the signs of both and invokes the custom modulo operator recursively. After calculating the remainder, it checks if it's negative and adds the divisor to make it positive. This ensures that the final result maintains the correct mathematical remainder. For instance, customMod(-1, 8) would return 7, while customMod(13, -8) would return -3.
Overall, this revised modulo operator effectively handles negative numbers and produces results that align with mathematical modulo expectations.
The above is the detailed content of How to Handle Negative Numbers in Modulo Operations in C/C /Obj-C?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


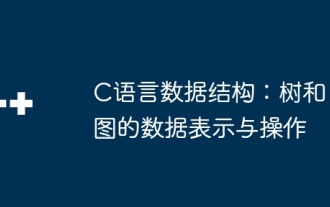
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
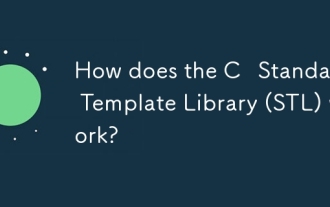
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
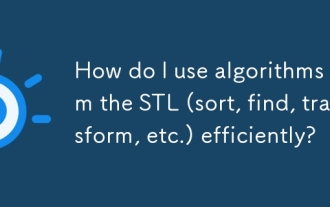
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
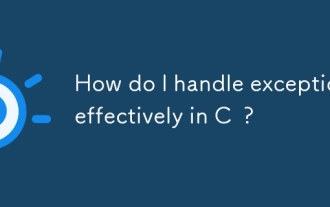
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
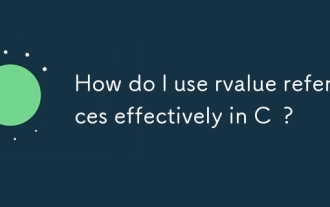
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
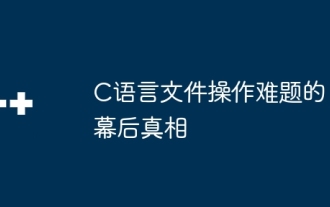
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
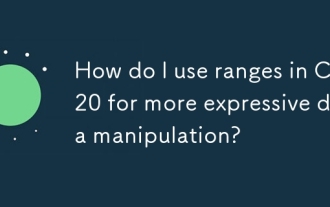
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
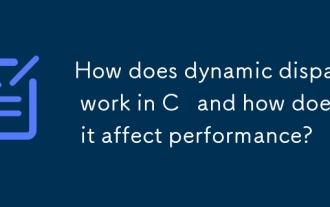
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
