How to Bind Multiple Key Press Events in Turtle Graphics?
Binding Multiple Key Press Events in Turtle Graphics
Coordinating user input from multiple key presses is crucial in many interactive applications, including games. In Turtle graphics, connecting the dots based on specific key combinations is a common requirement.
To efficiently handle multiple key presses together, the following approach has been widely used:
Using win.onkeypress()
The win.onkeypress() method in Turtle provides a straightforward way to bind key press events to specific functions. By registering event listeners for each individual key, we can execute desired actions when the corresponding keys are pressed.
For instance, consider a scenario where we want our Turtle to move in different directions based on key combinations. We can register event listeners for 'Up,' 'Down,' 'Left,' and 'Right' keys, and define separate functions to handle each movement.
<code class="python">import turtle flynn = turtle.Turtle() win = turtle.Screen() win.listen() def Up(): flynn.setheading(90) flynn.forward(25) def Down(): flynn.setheading(270) flynn.forward(20) def Left(): flynn.setheading(180) flynn.forward(20) def Right(): flynn.setheading(0) flynn.forward(20) win.onkeypress(Up, "Up") win.onkeypress(Down, "Down") win.onkeypress(Left, "Left") win.onkeypress(Right, "Right")</code>
With this code, when the user presses the 'Up' key, the Turtle will move 25 units in the north direction. Similarly, the Turtle will respond to 'Down,' 'Left,' and 'Right' key presses.
Handling Combinations
The above solution covers single key press events, but to handle combinations, such as 'Up' and 'Right' keys pressed together, we need to employ a more advanced approach.
Using the win.onkeypress() method exclusively for combination events can lead to timing issues and inconsistencies. Instead, we can utilize a timer mechanism to periodically check for the occurrence of any key press combination.
<code class="python">from turtle import Turtle, Screen win = Screen() flynn = Turtle('turtle') def process_events(): events = tuple(sorted(key_events)) if events and events in key_event_handlers: (key_event_handlers[events])() key_events.clear() win.ontimer(process_events, 200) def Up(): key_events.add('UP') def Down(): key_events.add('DOWN') def Left(): key_events.add('LEFT') def Right(): key_events.add('RIGHT') def move_up(): flynn.setheading(90) flynn.forward(25) def move_down(): flynn.setheading(270) flynn.forward(20) def move_left(): flynn.setheading(180) flynn.forward(20) def move_right(): flynn.setheading(0) flynn.forward(20) def move_up_right(): flynn.setheading(45) flynn.forward(20) def move_down_right(): flynn.setheading(-45) flynn.forward(20) def move_up_left(): flynn.setheading(135) flynn.forward(20) def move_down_left(): flynn.setheading(225) flynn.forward(20) key_event_handlers = { \ ('UP',): move_up, \ ('DOWN',): move_down, \ ('LEFT',): move_left, \ ('RIGHT',): move_right, \ ('RIGHT', 'UP'): move_up_right, \ ('DOWN', 'RIGHT'): move_down_right, \ ('LEFT', 'UP'): move_up_left, \ ('DOWN', 'LEFT'): move_down_left, \ } key_events = set() win.onkey(Up, "Up") win.onkey(Down, "Down") win.onkey(Left, "Left") win.onkey(Right, "Right") win.listen() process_events() win.mainloop()</code>
This approach maintains a list of key events in the key_events set, and each time the timer function is executed, it checks if any combinations are present in the key_event_handlers dictionary. If a combination is found, the corresponding handler function is executed, resulting in the Turtle performing the desired action.
The above is the detailed content of How to Bind Multiple Key Press Events in Turtle Graphics?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










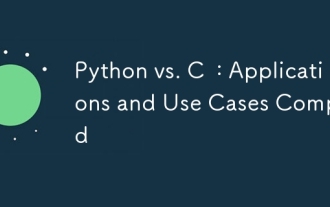
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
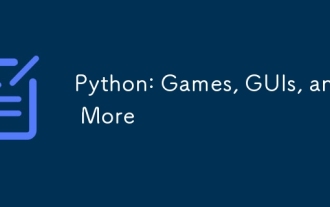
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
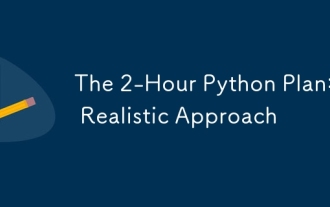
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
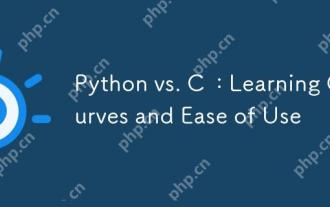
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
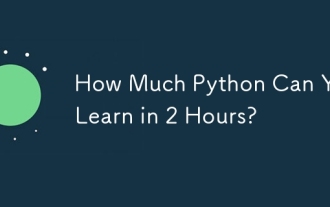
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
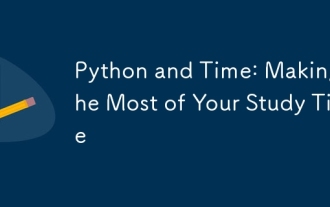
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
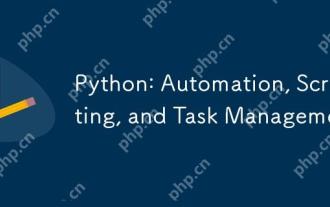
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
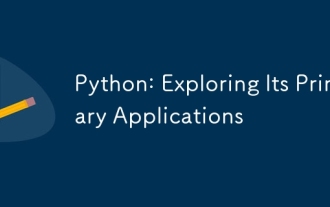
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
