


How Can We Defer the Evaluation of F-Strings Beyond the Immediate Code Context?
Nov 03, 2024 am 01:56 AMOvercoming the Evaluation Quandary in F-Strings
When composing files using template strings, the conciseness of f-strings is highly appealing. However, difficulties emerge when template definitions reside outside the immediate code context. How can we defer the evaluation of f-strings, eliminating the need for the format(**locals()) call?
Enter fstr(), a powerful function that solves this dilemma. Here's how it works:
<code class="python">def fstr(template): return eval(f'f&quot;&quot;&quot;{template}&quot;&quot;&quot;')</code>
To use this function, simply call fstr() on the desired template:
<code class="python">template_a = "The current name is {name}" names = ["foo", "bar"] for name in names: print(fstr(template_a)) # Output: # The current name is foo # The current name is bar</code>
Crucially, unlike other proposed solutions, fstr() allows for more complex expressions within the template, including function calls and attribute access:
<code class="python">template_b = "The current name is {name.upper() * 2}" for name in names: print(fstr(template_b)) # Output: # The current name is FOOFOO # The current name is BARBAR</code>
With this solution, you can effectively postpone the evaluation of f-strings, preserving their powerful capabilities for succinct and dynamic template handling.
The above is the detailed content of How Can We Defer the Evaluation of F-Strings Beyond the Immediate Code Context?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
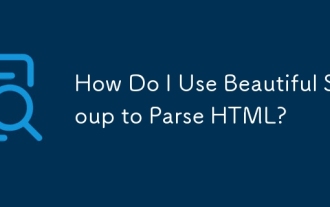
How Do I Use Beautiful Soup to Parse HTML?
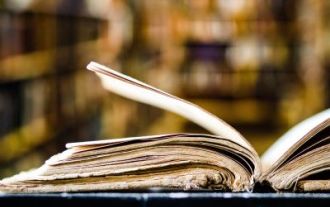
How to Use Python to Find the Zipf Distribution of a Text File
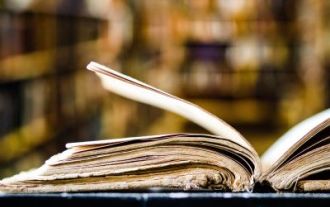
How to Work With PDF Documents Using Python
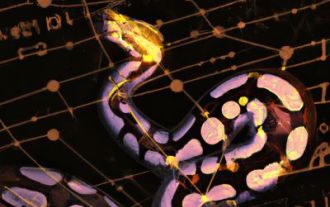
How to Cache Using Redis in Django Applications
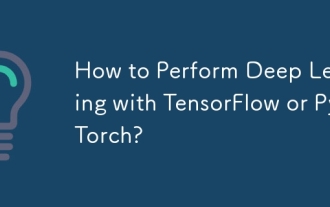
How to Perform Deep Learning with TensorFlow or PyTorch?
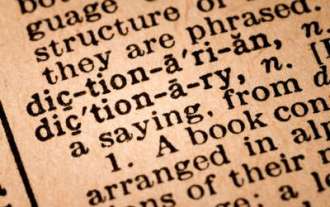
Introducing the Natural Language Toolkit (NLTK)
