


How Can I Query Data With Unknown Column Types in Go\'s SQL Package?
Exploring Ad Hoc Queries in Go's SQL Package
While the documentation suggests that querying data in Go using the SQL package requires knowing column count and types at compile-time, this is not strictly true. The sql.Rows type offers a solution for flexible and ad hoc SQL queries.
Dynamic Column Metadata Retrieval
The Columns method in sql.Rows provides a list of the result column names. This allows you to dynamically determine the number of columns returned by an arbitrary query.
Scanning Unknown Data Types
The Scan method supports scanning values of unknown types into either a raw byte slice (*[]byte) or an interface{} value. This enables you to retrieve column data without pre-defining its type.
Working with Ad Hoc Queries
Combining these techniques, you can execute ad hoc queries and retrieve data into a slice of interface{} values:
<code class="go">columnNames, err := rows.Columns() if err != nil { // Handle error } columns := make([]interface{}, len(columnNames)) columnPointers := make([]interface{}, len(columnNames)) for i := 0; i < len(columnNames); i++ { columnPointers[i] = &columns[i] } if err := rows.Scan(columnPointers...); err != nil { // Handle error }</code>
After this, the columns slice will contain the decoded values for all columns in the result row. By leveraging the Columns and Scan methods, you can effectively handle ad hoc queries in Go's SQL package.
The above is the detailed content of How Can I Query Data With Unknown Column Types in Go\'s SQL Package?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


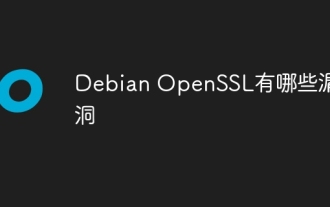
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
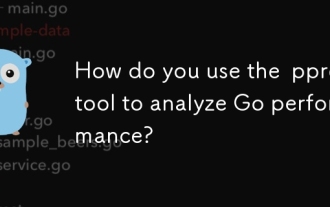
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
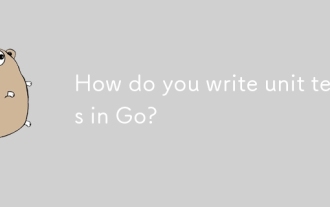
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
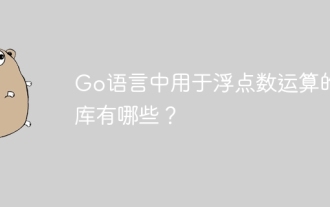
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
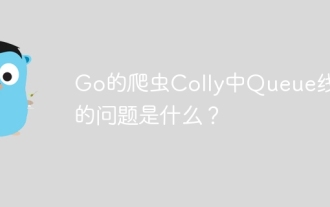
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
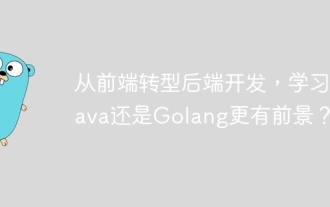
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
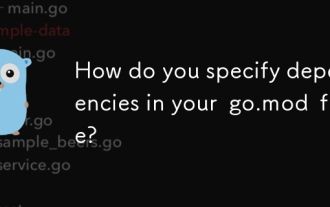
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
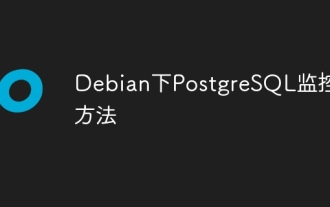
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
