How to Access ViewPager Fragment Methods from an Activity?
Nov 03, 2024 am 05:05 AMAccess ViewPager Fragment Method from Activity
Many mobile applications utilize fragments, self-contained components representing a modular screen section. Managing multiple fragments using a view pager grants smooth navigation and page animations. Occasionally, developers face the need to perform specific actions within a fragment in response to external events, such as a user swipe on the view pager. However, implementing this functionality can encounter certain challenges.
Initial Approaches and Limitations
Initially, an attempt was made to detect fragment visibility by overriding the setUserVisibleHint method. While this approach worked, it introduced a noticeable lag during fragment transitions.
An alternative approach involved utilizing OnPageChangeListener, a listener registered to the view pager, which provided more control over the timing of fragment actions. However, attempting to execute a method within a fragment from the OnPageChangeListener resulted in a NullPointerException.
Solution
The solution lies in maintaining a reference to each fragment instance within the pager adapter. The ViewPager provides a method called instantiateItem that can be overridden to store fragment references in an array or collection. During the onPageSelected event, you can access the desired fragment instance from the adapter and perform necessary actions, such as initiating a network request.
Example Code (Java)
<code class="java">public class PagerAdapter extends FragmentPagerAdapter { private Fragment[] fragments; public PagerAdapter(FragmentManager fm, Context context) { super(fm); } @Override public Object instantiateItem(ViewGroup container, int position) { Fragment createdFragment = (Fragment) super.instantiateItem(container, position); fragments[position] = createdFragment; return createdFragment; } } // Within your activity that hosts view pager: onPageChangeListener = new ViewPager.OnPageChangeListener() { @Override public void onPageSelected(int position) { // Assuming you named your fragment FragmentTwo Fragment frag = adapter.fragments[position]; if (frag != null && frag instanceof FragmentTwo) { ((FragmentTwo) frag).sendGetRequest(); } } };</code>
Kotlin Equivalent (with Kotlin Extensions and ViewPager2)
<code class="kotlin">viewPager.registerOnPageChangeCallback( object : ViewPager2.OnPageChangeCallback() { override fun onPageSelected(position: Int) { val frag = mAdapter.fragments[position] if (frag is FragmentTwo) { frag.sendGetRequest() } } } )</code>
Conclusion
By implementing this approach, you can effectively communicate with fragments from your hosting activity, enabling seamless integration and enhanced user experiences within your mobile applications.
The above is the detailed content of How to Access ViewPager Fragment Methods from an Activity?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
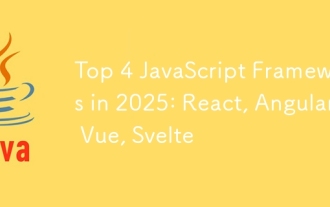
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
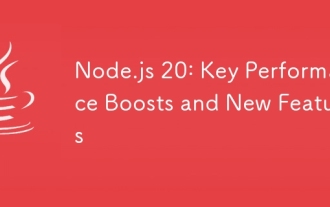
Node.js 20: Key Performance Boosts and New Features
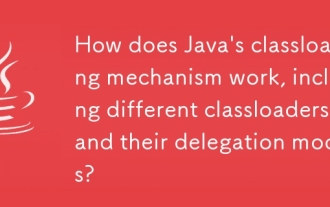
How does Java's classloading mechanism work, including different classloaders and their delegation models?
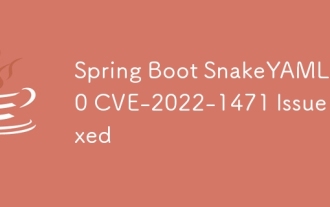
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
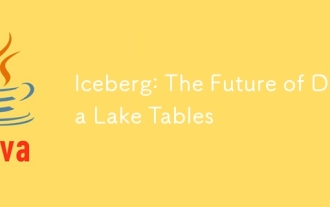
Iceberg: The Future of Data Lake Tables
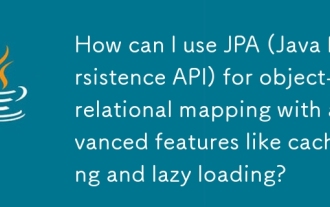
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
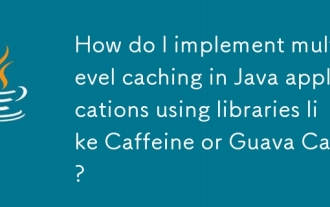
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
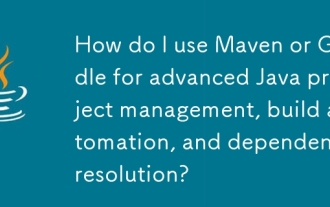
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
