When to Embed a Pointer to a Struct vs. the Struct Itself in Go?
Embedding a Pointer to a Struct vs. Embedding the Struct Itself
In the given scenario, where a struct type A is used as a pointer and solely contains pointer receivers, there exists a distinction between embedding a struct type B as B versus *B.
Zero Value Behavior
The most apparent difference lies in the zero values of the resultant structures. When embedding B as is, the zero value of type A will include an embedded object of type B, which also holds its zero value. This enables seamless usage of the embedded field without prior initialization:
type B struct { X int } func (b *B) Print() { fmt.Printf("%d\n", b.X) } type AObj struct { B } var aObj AObj aObj.Print() // prints 0
On the other hand, embedding *B results in a zero value with a nil pointer, preventing direct field access:
type APtr struct { *B } var aPtr APtr aPtr.Print() // panics
Field Copying
When creating new objects of type A, the behavior of field copying aligns with intuition. Embedding B directly creates a copy of the embedded object within the new object, preserving independence:
aObj2 := aObj aObj.X = 1 aObj2.Print() // prints 0, due to a separate instance of B
Conversely, embedding *B creates a copy of the pointer rather than the underlying concrete object. This means that both objects share the same B instance, impacting field modifications:
aPtr.B = &B{} aPtr2 := aPtr aPtr.X = 1 aPtr2.Print() // prints 1, as both objects point to the same B
In essence, embedding B creates distinct instances of the contained struct, while embedding *B shares a single concrete instance across multiple embedded structures. This distinction affects both the zero value semantics and the behavior of field copying.
The above is the detailed content of When to Embed a Pointer to a Struct vs. the Struct Itself in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










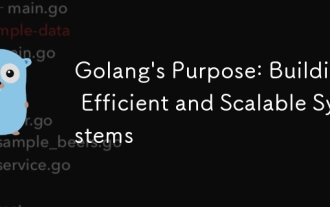
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
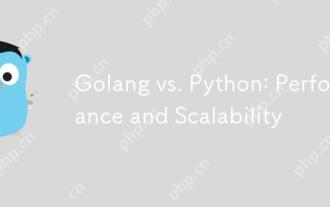
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
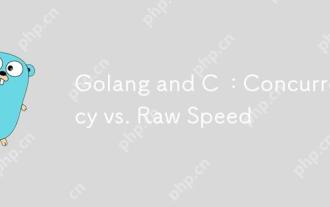
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
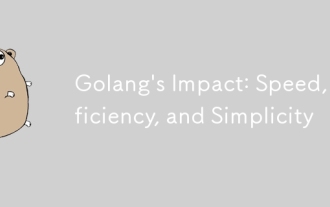
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
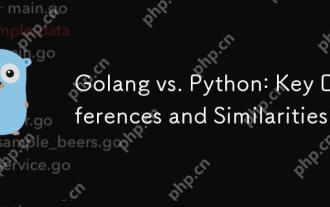
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
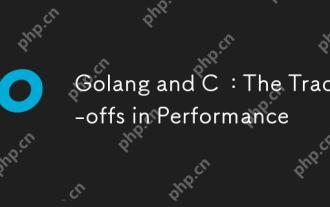
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
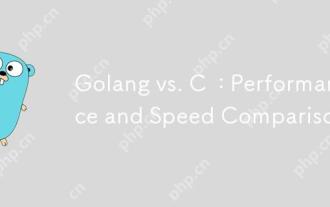
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
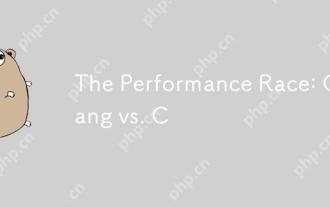
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
