How to Preserve Line Breaks When Converting HTML to Plain Text with Jsoup?
Preserving Line Breaks When Converting HTML to Plain Text with Jsoup
When converting HTML content to plaintext, it's essential to maintain line breaks for readability. By default, Jsoup's text() method strips line breaks, potentially disrupting the formatting of your output. Fortunately, there's a workaround that leverages the getWholeText() method to preserve line breaks.
Using getWholeText() to Preserve Line Breaks
The getWholeText() method in Jsoup returns the entire text content of a document, including line breaks. To utilize this method, you can follow these steps:
-
Parse your HTML string using Jsoup:
<code class="java">Document doc = Jsoup.parse(htmlString);</code>
Copy after login -
Iterate over the document's elements and extract text:
<code class="java">for (Element element : doc.getAllElements()) { text += element.getWholeText().trim(); if (element.tagName().equals("br")) { text += "\n"; } }</code>
Copy after login
By adding a line break after each
tag, you can ensure that line breaks are preserved in your output text.
Advanced Solution: br2nl() Method
The above solution works effectively but can be improved by incorporating the following utility method:
<code class="java">public static String br2nl(String html) { if (html == null) { return html; } Document document = Jsoup.parse(html); document.outputSettings(new Document.OutputSettings().prettyPrint(false)); document.select("br").append("\n"); document.select("p").prepend("\n\n"); String s = document.html().replaceAll("\\n", "\n"); return Jsoup.clean(s, "", Whitelist.none(), new Document.OutputSettings().prettyPrint(false)); }</code>
This method not only preserves line breaks from
and
tags but also ensures that newlines in the original HTML are retained. It does this by selectively prepending and appending line breaks to HTML elements and then performing a regex substitution to replace the escaped line breaks with actual newlines.
The above is the detailed content of How to Preserve Line Breaks When Converting HTML to Plain Text with Jsoup?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










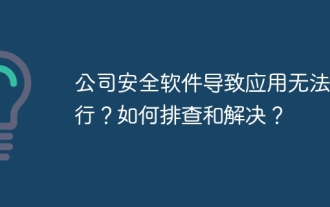
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
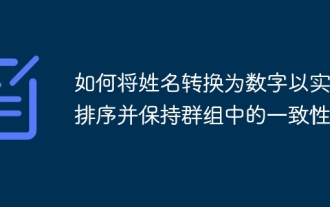
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
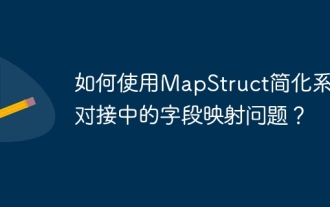
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
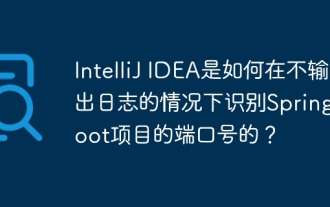
Start Spring using IntelliJIDEAUltimate version...
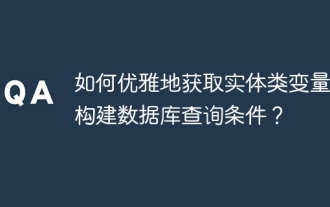
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
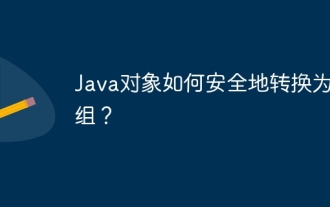
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
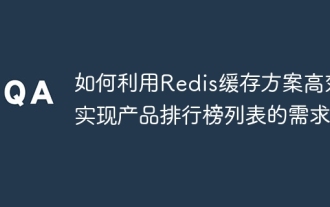
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
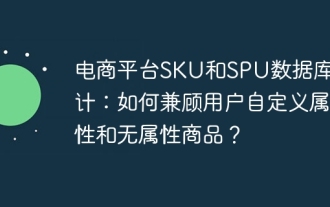
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
