


How to Seamlessly Transition from MySQL to MySQLi: A Step-by-Step Guide for Developers
Transition from MySQL to MySQLi: A Comprehensive Guide
As MySQL nears its deprecation, it's imperative for developers to upgrade to its successor, MySQLi. However, the transition can be daunting, especially for those accustomed to the MySQL syntax. This article provides a detailed guide on how to convert MySQL code to MySQLi, focusing on database querying techniques.
Querying with MySQLi
To convert MySQL querying syntax to MySQLi, follow these steps:
-
Instantiate a MySQLi connection:
<code class="php">$connection = mysqli_connect("host", "username", "password", "database");</code>
Copy after login -
Prepare the query:
<code class="php">$sql_follows = "SELECT * FROM friends WHERE user1_id=? AND status=2 OR user2_id=? AND status=2";</code>
Copy after loginNote the use of placeholders (?) for values that will be bound later.
-
Bind the values:
<code class="php">$stmt = mysqli_prepare($connection, $sql_follows); mysqli_stmt_bind_param($stmt, "ii", $_SESSION['id'], $_SESSION['id']);</code>
Copy after login -
Execute the query:
<code class="php">mysqli_stmt_execute($stmt);</code>
Copy after login -
Check for results:
<code class="php">$result = mysqli_stmt_get_result($stmt); if (mysqli_num_rows($result) > 0) { // Query successful and has results } else { // Query successful but has no results }</code>
Copy after login
Converter Tools and Shim Library
For those facing challenges in converting their code, there are several resources available:
- MySQLConverterTool: This tool automates the conversion process. However, its generated code may require further refinement.
- MySQL Shim Library: This library allows developers to use the MySQL syntax while working with MySQLi.
Further Considerations
- Ensure that the server supports MySQLi before deploying your code.
- Replace deprecated functions with their MySQLi counterparts.
- Check for any exceptions or errors during the transition process.
- Thoroughly test your converted code to ensure its correct functionality.
By following these guidelines, you can successfully upgrade your code from MySQL to MySQLi and continue to work with database queries efficiently.
The above is the detailed content of How to Seamlessly Transition from MySQL to MySQLi: A Step-by-Step Guide for Developers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










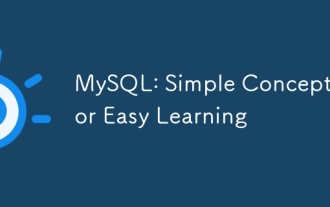
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
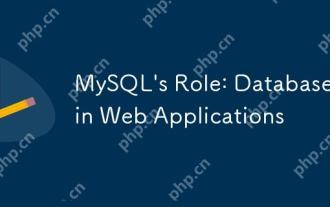
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
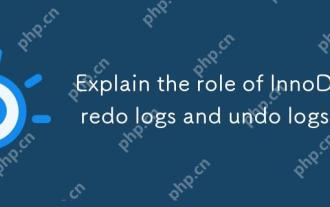
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
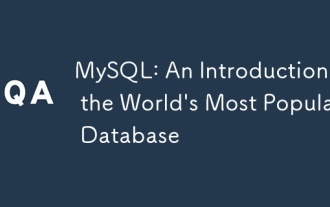
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
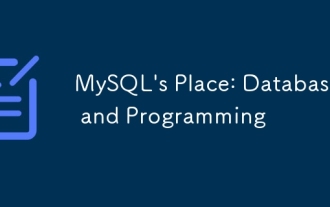
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
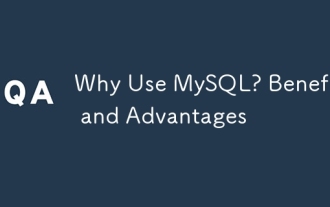
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
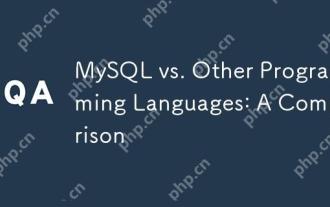
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
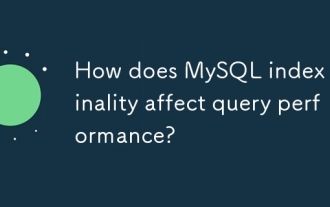
MySQL index cardinality has a significant impact on query performance: 1. High cardinality index can more effectively narrow the data range and improve query efficiency; 2. Low cardinality index may lead to full table scanning and reduce query performance; 3. In joint index, high cardinality sequences should be placed in front to optimize query.
