


How to Merge Multiple Associative Arrays and Sum Values of Shared Keys in PHP?
How to Merge Multiple Associative Arrays and Sum Values of Shared Keys
The Challenge
As you may have encountered, the built-in array_merge() function in PHP overwrites the values of shared keys when combining multiple arrays. This can be problematic when you need to merge arrays and sum the values of shared keys instead.
A Solution
To address this challenge, we can create a custom function or leverage array operations to achieve our desired behavior. Let's explore a few possible approaches:
Using a Custom Function
<code class="php">function array_sum_identical_keys() { $arrays = func_get_args(); $keys = array_keys(array_reduce($arrays, function ($keys, $arr) { return $keys + $arr; }, array())); $sums = array(); foreach ($keys as $key) { $sums[$key] = array_reduce($arrays, function ($sum, $arr) use ($key) { return $sum + @$arr[$key]; }); } return $sums; }</code>
This function accepts an arbitrary number of arrays as arguments and returns an associative array with the combined keys and summed values.
Using Error Suppression
<code class="php">$sums = array(); foreach (array_keys($a1 + $a2) as $key) { $sums[$key] = @($a1[$key] + $a2[$key]); }</code>
This technique leverages the error suppression operator (@) to check for the existence of keys in both arrays before performing the addition.
Using Array Mappings
<code class="php">$keys = array_fill_keys(array_keys($a1 + $a2), 0); $sums = array_map(function ($a1, $a2) { return $a1 + $a2; }, array_merge($keys, $a1), array_merge($keys, $a2));</code>
This approach creates a mapping using array_fill_keys() and then uses array_map() to add the values associated with the shared keys.
Combining Solutions
<code class="php">$sums = array_fill_keys(array_keys($a1 + $a2), 0); array_walk($sums, function (&$value, $key, $arrs) { $value = @($arrs[0][$key] + $arrs[1][$key]); }, array($a1, $a2));</code>
This combination combines the error suppression technique with array_walk() to iterate over the keys and perform the addition.
Conclusion
Depending on your specific requirements, you can choose the most suitable method to merge multiple arrays and sum the values of shared keys. Whether it's using a custom function, error suppression, array mappings, or a combination thereof, these solutions provide a concise and effective way to achieve the desired result.
The above is the detailed content of How to Merge Multiple Associative Arrays and Sum Values of Shared Keys in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










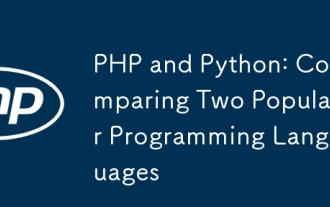
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
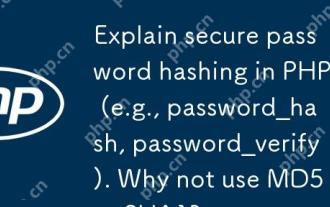
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
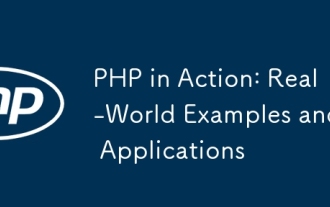
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
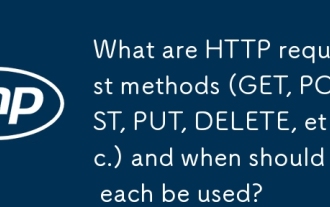
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
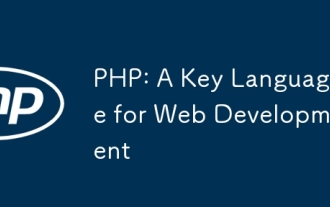
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
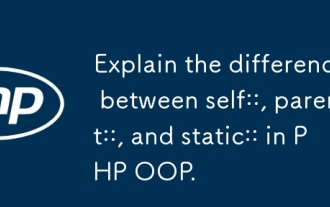
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
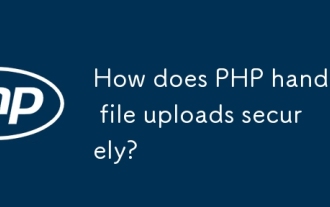
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
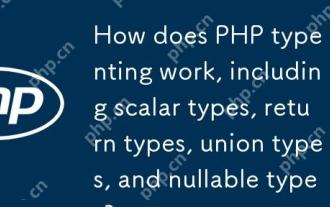
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
