


How can I optimize image cropping in PHP for large images while preserving the aspect ratio?
Image Cropping in PHP: Optimization for Large Images and Aspect Ratio Preservation
The code snippet provided effectively crops images, but the results may deteriorate when applied to larger images. To address this issue, we will explore an alternative approach that involves resizing the original image before cropping to achieve consistent and optimal results.
Resizing for Aspect Ratio Preservation
Before cropping an image, it is essential to maintain its aspect ratio to avoid distortion. The aspect ratio is the proportion of the image's width to its height. By resizing the image so that the smaller side matches the desired crop dimensions, we can preserve the original aspect ratio.
Code Implementation
To implement image resizing and cropping, we will modify the following portion of the provided code:
<code class="php">$image = imagecreatefromjpeg($_GET['src']); $filename = 'images/cropped_whatever.jpg'; $thumb_width = 200; $thumb_height = 150; $width = imagesx($image); $height = imagesy($image); $original_aspect = $width / $height; $thumb_aspect = $thumb_width / $thumb_height; if ( $original_aspect >= $thumb_aspect ) { // If image is wider than thumbnail (in aspect ratio sense) $new_height = $thumb_height; $new_width = $width / ($height / $thumb_height); } else { // If the thumbnail is wider than the image $new_width = $thumb_width; $new_height = $height / ($width / $thumb_width); } $thumb = imagecreatetruecolor( $thumb_width, $thumb_height ); // Resize and crop imagecopyresampled($thumb, $image, 0 - ($new_width - $thumb_width) / 2, // Center the image horizontally 0 - ($new_height - $thumb_height) / 2, // Center the image vertically 0, 0, $new_width, $new_height, $width, $height); imagejpeg($thumb, $filename, 80);</code>
Explanation
- We calculate the original aspect ratio (original_aspect) and the target aspect ratio (thumb_aspect) to determine the new dimensions of the image.
- We check if the original image is wider than the thumbnail in terms of aspect ratio.
- If the original image is wider, we set the new height to match the thumbnail height and calculate the new width proportionally.
- If the thumbnail is wider, we set the new width to match the thumbnail width and calculate the new height proportionally.
- We create a new image ($thumb) with the desired thumbnail dimensions.
- We use imagecopyresampled() to resize and crop the original image into the new thumbnail, maintaining the aspect ratio.
- Finally, we save the cropped thumbnail to the specified file.
By following this approach, we can effectively crop larger images and preserve the aspect ratio, delivering consistent and high-quality results.
The above is the detailed content of How can I optimize image cropping in PHP for large images while preserving the aspect ratio?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










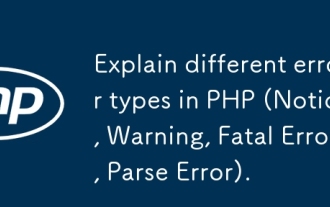
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
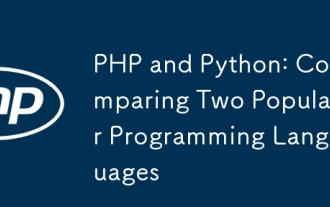
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
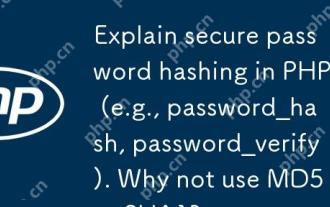
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
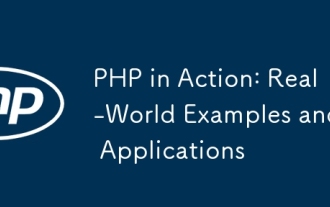
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
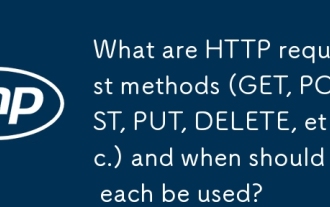
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
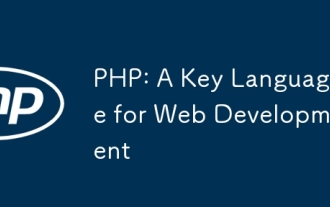
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
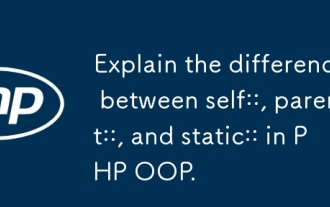
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
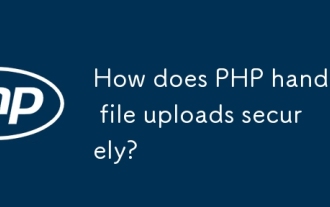
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
