Why Can\'t I Create Arrays of Generic Classes in Java?
Creating Arrays of Generic Classes
When attempting to initialize an array of a generic class, such as ArrayList
Error Cause
Java does not support the direct creation of arrays of generic classes due to type safety concerns. If this functionality were allowed, it could lead to potential type errors, as illustrated in the following example:
<code class="java">List<String>[] lsa = new List<String>[10]; // illegal Object[] oa = lsa; // OK because List<String> is a subtype of Object List<Integer> li = new ArrayList<Integer>(); li.add(new Integer(3)); oa[0] = li; String s = lsa[0].get(0);</code>
In this scenario, the oa array is assigned to the lsa array, but then an Integer value is added to the list at index 0. When retrieving the element at index 0 from lsa and attempting to cast it to String, a ClassCastException would occur.
Workaround
To circumvent this limitation, consider using a collection instead of an array. For instance:
<code class="java">public static ArrayList<List<MyObject>> a = new ArrayList<List<MyObject>>();</code>
Alternatively, an auxiliary class can be created to act as the array element type:
<code class="java">class MyObjectArrayList extends ArrayList<MyObject> { }</code>
<code class="java">MyObjectArrayList[] a = new MyObjectArrayList[2];</code>
By using a collection or auxiliary class, the Java compiler can ensure type safety and prevent potential errors.
The above is the detailed content of Why Can\'t I Create Arrays of Generic Classes in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










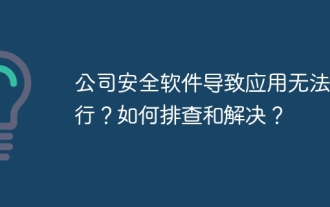
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
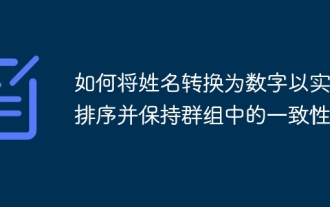
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
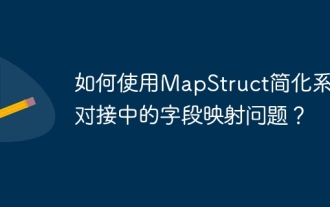
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
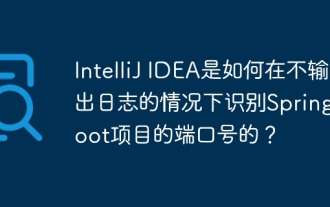
Start Spring using IntelliJIDEAUltimate version...
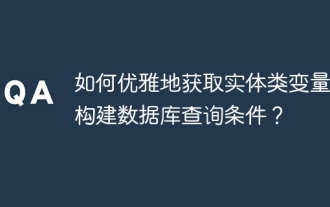
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
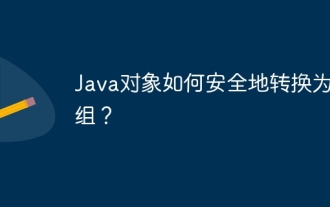
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
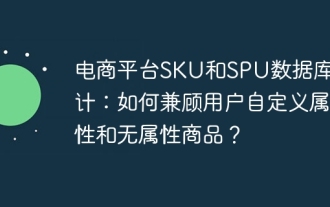
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
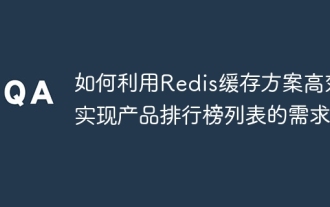
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
