


Why Can\'t I Delete a File in Java Even with Read, Write, and Execute Permissions?
File Deletion Failure Despite Existing File with Read, Write, and Execute Permissions
In Java, attempting to delete a file using file.delete() may encounter unexpected failures, even if the file exists and has appropriate read, write, and execute permissions as determined by file.exists(), file.canRead(), file.canWrite(), and file.canExecute().
To resolve this issue, it is crucial to ensure that all resources associated with the file are properly closed and released. A common cause of deletion failures is the retention of a file handle or stream that prevents the operating system from removing the file.
Consider the following code snippet:
<code class="java">private void deleteFile(File file) { boolean result = file.delete(); if (!result) { throw new IOException("File deletion failed"); } }</code>
In this example, the deleteFile method attempts to delete a file but throws an exception if the deletion fails. However, if the file is still open or otherwise referenced by an active resource, the deletion will fail.
To address this issue, it is recommended to explicitly close any streams or file handles that have been opened to access the file. Additionally, Java's garbage collection system can be encouraged to release resources and finalize objects by calling System.gc(). Here is an example of how this can be implemented:
<code class="java">private void deleteFile(File file) { try { if (file.exists()) { FileInputStream in = new FileInputStream(file); in.close(); } } catch (IOException e) { // Handle file access failure } finally { System.gc(); boolean result = file.delete(); if (!result) { throw new IOException("File deletion failed"); } } }</code>
By explicitly closing the file handle and calling System.gc(), it is more likely that all resources associated with the file will be released, allowing the deletion to succeed. It is important to note that System.gc() is only a suggestion to the garbage collector and does not guarantee immediate release of resources.
The above is the detailed content of Why Can\'t I Delete a File in Java Even with Read, Write, and Execute Permissions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










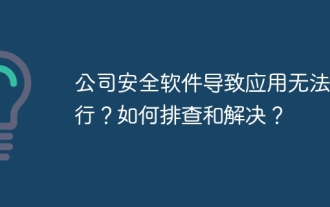
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
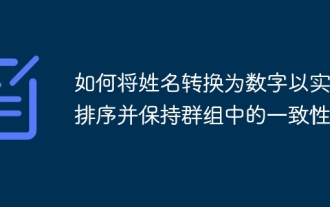
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
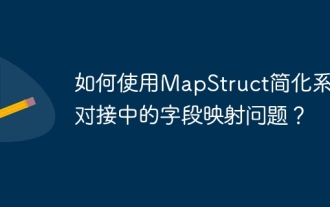
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
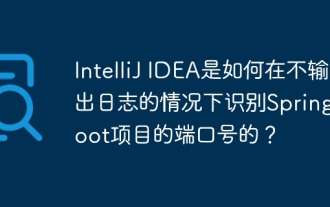
Start Spring using IntelliJIDEAUltimate version...
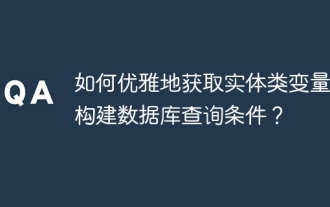
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
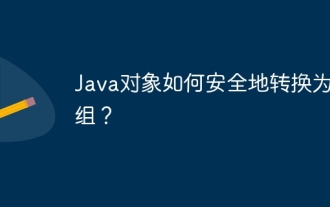
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
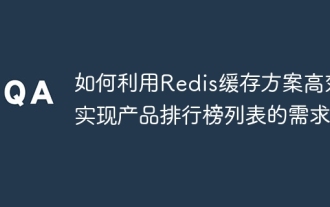
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
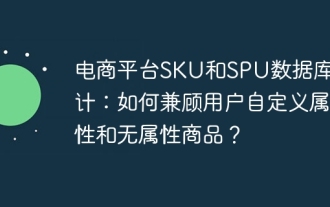
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
