How Do You Interact with C Classes from Swift?
Nov 03, 2024 pm 09:58 PMInteracting with C Classes from Swift: A Comprehensive Guide
Interfacing with C code from Swift can be a valuable solution for utilizing existing libraries and reducing code duplication. However, it poses a specific challenge when dealing with C classes rather than functions. This article provides a detailed guide on how to instantiate and manipulate C classes from within Swift.
Bridging Header for C Functions
Before delving into C class interaction, let's review the process of bridging to C functions:
Define a bridging header with "C" functions to expose C functionality to Swift:
<code class="c">#define ImageReader_hpp #ifdef __cplusplus extern "C" { #endif const char *hexdump(char *filename); const char *imageType(char *filename); #ifdef __cplusplus } #endif</code>
Swift code can then directly call these functions:
<code class="swift">let type = String.fromCString(imageType(filename)) let dump = String.fromCString(hexdump(filename))</code>
Interacting with C Classes from Swift
To work with C classes in Swift, the approach is slightly different:
Create C Wrapper Functions
For each C class, create C wrapper functions that interface with its functionality:
<code class="c++">MBR *initialize(char *filename) { return new MBR(filename); } const char *hexdump(MBR *object) { static char retval[2048]; strcpy(retval, object->hexdump()); return retval; }</code>
Define Bridge Header for Wrapper Functions
Include the wrapper functions in a bridging header:
<code class="c">#define ImageReader_hpp #ifdef __cplusplus extern "C" { #endif MBR *initialize(char *filename); const char *hexdump(MBR *object); #ifdef __cplusplus } #endif</code>
Instantiate and Interact from Swift
In Swift, instantiate the C class using the initializer wrapper function:
<code class="swift">let cppObject = initialize(filename)</code>
Access class methods using the wrapper functions:
<code class="swift">let type = String.fromCString(hexdump(cppObject))</code>
Encapsulation for Cleaner Code
To improve code readability, encapsulate the bridging code in a Swift class, removing the need for direct interaction with C pointers:
<code class="swift">class MBRWrapper { private var _object: MBR * init(filename: String) { _object = initialize(filename) } func hexdump() -> String { return String.fromCString(hexdump(_object)) } }</code>
This abstraction allows you to work with C objects like native Swift objects, hiding the underlying bridging mechanism.
The above is the detailed content of How Do You Interact with C Classes from Swift?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
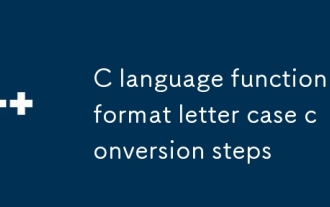
C language function format letter case conversion steps
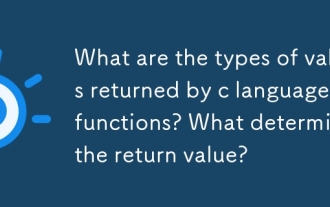
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
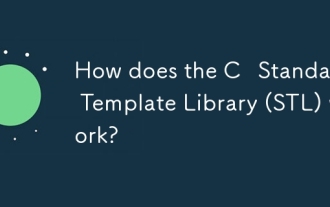
How does the C Standard Template Library (STL) work?

Where is the return value of the c language function stored in memory?
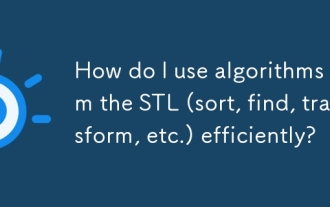
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
