How to Effectively Parse JSON Arrays with Gson: Avoiding Common Pitfalls?
Understanding JSON Array Parsing with Gson
Question:
How do I parse JSON arrays using Gson while avoiding common pitfalls?
Response:
To effectively parse JSON arrays with Gson, consider the following guidelines:
Avoiding Unnecessary Wrapping
Json arrays can be directly parsed without additional wrappers. The PostEntity class introduced in the original post is not necessary. Here's an example:
1 2 3 4 |
|
Direct JSON Input
Avoid using JSONObject to convert the JSON string to a string before parsing. Gson can parse JSON strings directly. This eliminates unnecessary overhead.
Troubleshooting Parsing Issues
If parsing fails without errors or warnings, the issue may lie in the JSON structure. Verify that the array is formatted correctly. For instance, ensure that it follows the sample JSON output provided in the original post.
Conclusion
By avoiding unnecessary wrapping, utilizing direct JSON input, and troubleshooting potential formatting issues, you can efficiently parse JSON arrays with Gson.
The above is the detailed content of How to Effectively Parse JSON Arrays with Gson: Avoiding Common Pitfalls?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
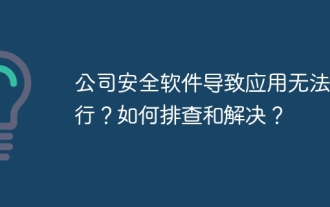
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
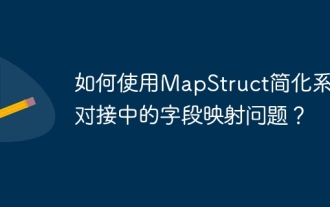
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
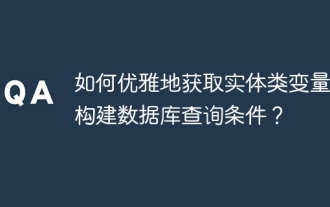
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
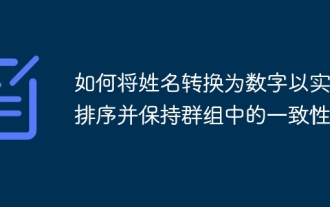
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
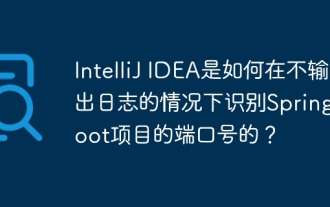
Start Spring using IntelliJIDEAUltimate version...
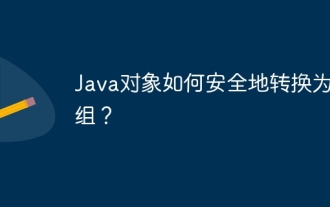
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
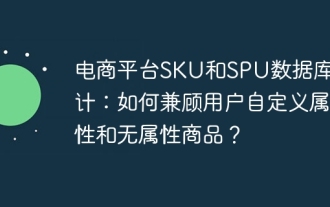
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
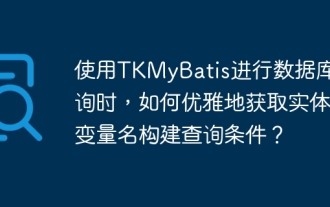
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
