Can You Compare Iterators from Different Containers in C ?
Comparing Iterators from Different Containers: A Cautionary Tale
In C , iterators provide a powerful mechanism for traversing collections. However, it is important to be aware of the limitations when using iterators from different containers.
The question of whether it is legal to compare iterators from different containers arises frequently. Consider the following example:
<code class="cpp">std::vector<int> foo; std::vector<int> bar; std::cout << (foo.begin() == bar.begin());</code>
This expression may seem harmless at first glance, but it actually yields undefined behavior. According to the C 11 standard, iterators can only be compared if they refer to elements of the same sequence. Since foo and bar are two distinct vectors, their iterators are not comparable.
This behavior is further emphasized in LWG issue #446:
"The result of directly or indirectly evaluating any comparison function or the binary - operator with two iterator values as arguments that were obtained from two different ranges r1 and r2 (...) which are not subranges of one common range is undefined, unless explicitly described otherwise."
This restriction has significant implications for implementing custom iterators. If you plan to implement an operator== for your custom iterator, you must ensure that it only compares iterators that are within the same range.
Failing to adhere to this rule can lead to unexpected behavior and is ultimately detrimental to the reliability of your code. Therefore, it is crucial to keep in mind that comparing iterators from different containers is strictly prohibited in C .
The above is the detailed content of Can You Compare Iterators from Different Containers in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


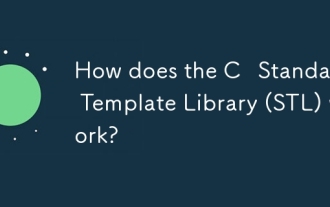
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
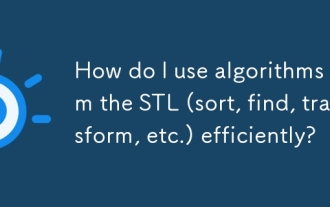
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
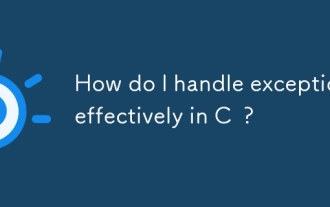
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
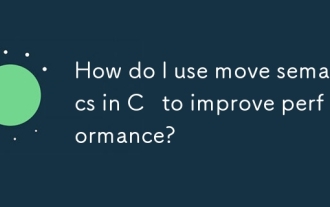
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
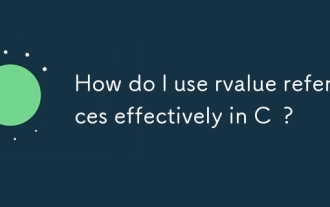
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
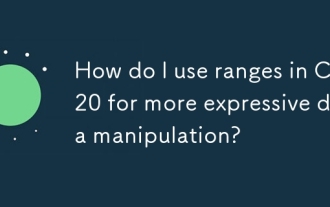
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
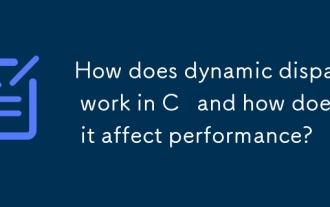
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
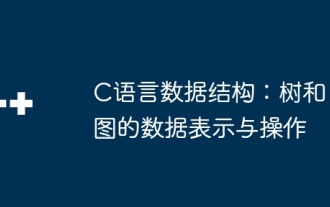
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
