


Enum Singleton in Java: Instance Method vs. Static Method - What\'s the Difference?
Single Instance Implementation Using Enum in Java: Understanding the Differences
Singleton patterns ensure that only a single instance of a class is created. One approach for implementing singletons in Java is utilizing enums. However, there are variations in this approach that raise questions about their differences and merits.
Let's explore the two scenarios you outlined:
Scenario 1: Using an Instance Method
<code class="java">public enum Elvis { INSTANCE; private int age; public int getAge() { return age; } }</code>
In this case, you can access the instance and its method as follows:
<code class="java">Elvis.INSTANCE.getAge();</code>
Scenario 2: Using a Static Method
<code class="java">public enum Elvis { INSTANCE; private int age; public static int getAge() { return INSTANCE.age; } }</code>
Here, you can access the instance's property through the static method:
<code class="java">Elvis.getAge();</code>
Differences and Considerations:
- Instance Binding: Scenario 1 allows direct binding to the instance (Elvis.INSTANCE), which is useful when properties of the object are required. Scenario 2, on the other hand, restricts binding to static methods.
- Property Accessibility: If the binding target searches for class properties, using Elvis.class with Scenario 1 will not locate the property unless explicitly coded. Scenario 2, however, ensures that the property is accessible through the static method.
Choosing the Approach:
Ultimately, the choice between scenarios depends on your specific needs:
- If an instance is required and properties need to be modified or accessed, Scenario 1 is more appropriate.
- If only static methods suffice, Scenario 2 is a simpler and more direct option. In cases where instance creation is not necessary or undesirable, this approach is preferred.
The above is the detailed content of Enum Singleton in Java: Instance Method vs. Static Method - What\'s the Difference?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










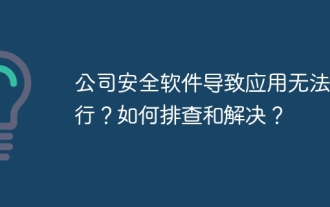
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
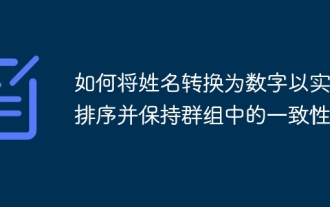
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
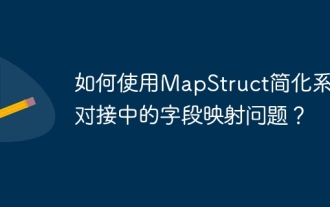
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
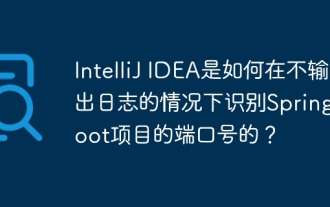
Start Spring using IntelliJIDEAUltimate version...
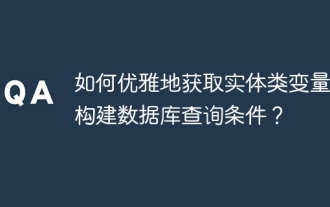
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
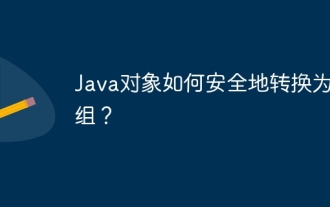
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
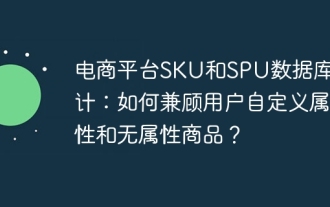
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
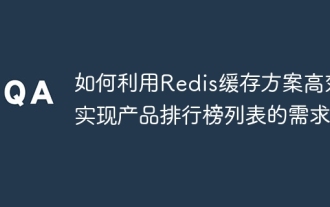
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
