


How to Extract a Specific Number of Words from a String in PHP?
Extracting the Initial Words of a String
Problem: Limit textual content by extracting only a specified number of words from a string.
Solution: Utilize the following approach:
Using implode() and array_slice()
<code class="php">implode(' ', array_slice(explode(' ', $sentence), 0, 10));</code>
- Split the string into an array of words using explode().
- Slice the array to retain only the desired number of words (in this case, 10).
- Join the sliced array back into a string using implode().
Using preg_match() for Enhanced Word-Breaking Customization
<code class="php">function get_words($sentence, $count = 10) { preg_match("/(?:\w+(?:\W+|$)){0,$count}/", $sentence, $matches); return $matches[0]; }</code>
- This function utilizes regular expressions to match groups of word characters (w ) that may be interspersed with non-word characters (W ) or the end of the line ($).
- The {0,$count} quantifier restricts matches to the desired number of words.
- The function returns the matching words as a single string.
UTF-8/Unicode Handling
PHP's built-in functions may not handle UTF-8/Unicode characters optimally. To address this:
- Replace w with 1 for word characters.
- Replace W with [s,.;?!] for non-word characters.
- s,.;?! ↩
The above is the detailed content of How to Extract a Specific Number of Words from a String in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
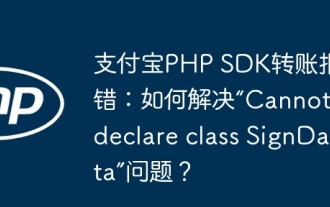
Alipay PHP...
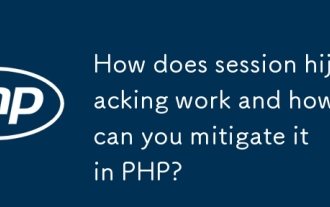
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
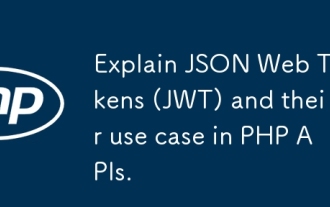
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
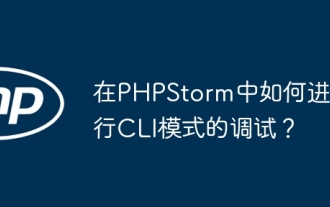
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
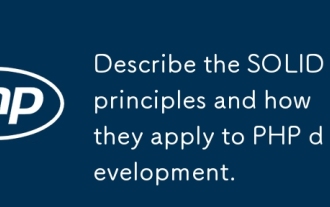
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
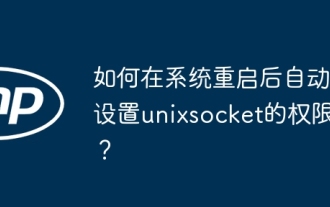
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
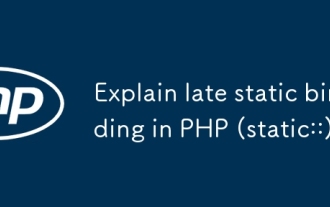
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
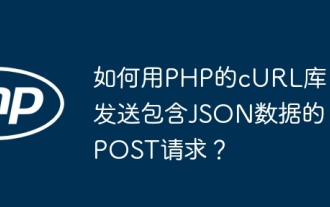
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
