How Can I Check if Three Values are Equal in Go?
Checking Equality of Three Values Elegantly
While the traditional approach with if a == b == c results in a syntax error, there are alternative methods to determine whether three values are equal.
Using a Clear and Concise Approach
The simplest solution remains:
1 2 3 |
|
This solution is straightforward and efficient, making comparisons on a per-pair basis.
Exploring Creative Solutions
Using a Map as a Set:
The len() function returns the number of unique keys in a map. By using a map with interface{} keys, we can check if all values are equal by comparing the map length to 1:
1 2 3 |
|
With Arrays:
Arrays are comparable, allowing us to compare multiple elements at once:
1 2 3 |
|
Using a Tricky Map:
We can index a map with a key that results in the comparison value:
1 2 3 |
|
With Anonymous Structs:
Structs are also comparable, so we can create an anonymous struct with the values and compare them:
1 2 3 |
|
With Slices:
To compare slices, we utilize the reflect.DeepEqual() function:
1 2 3 |
|
Using a Helper Function:
We can define a helper function to handle any number of values:
1 2 3 4 5 6 7 8 9 10 11 |
|
The above is the detailed content of How Can I Check if Three Values are Equal in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
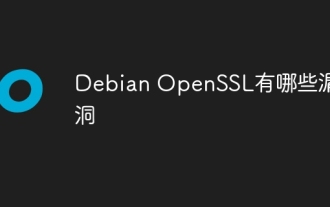
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
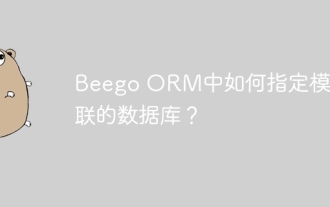
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
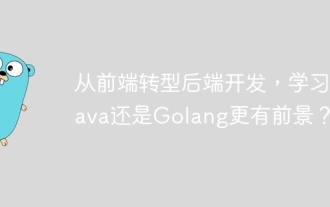
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
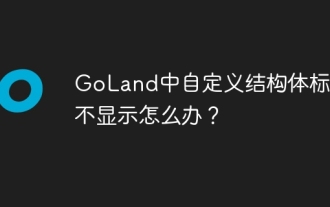
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
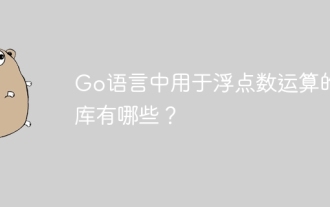
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
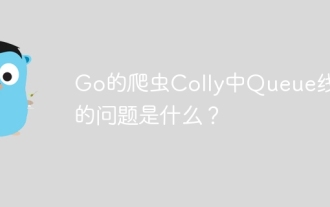
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
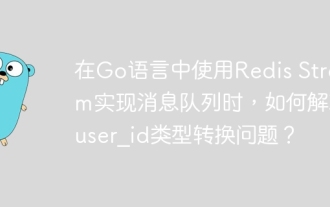
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...

This article introduces how to configure MongoDB on Debian system to achieve automatic expansion. The main steps include setting up the MongoDB replica set and disk space monitoring. 1. MongoDB installation First, make sure that MongoDB is installed on the Debian system. Install using the following command: sudoaptupdatesudoaptinstall-ymongodb-org 2. Configuring MongoDB replica set MongoDB replica set ensures high availability and data redundancy, which is the basis for achieving automatic capacity expansion. Start MongoDB service: sudosystemctlstartmongodsudosys
