


How can I mock `http.Request.FormFile` in Go for testing web endpoints?
Testing Go: Mocking Request.FormFile
In the process of testing Go web endpoints, one may encounter the challenge of mocking the http.Request.FormFile field. This field represents uploaded files within a request and is essential for testing endpoint functionality.
To address this, one might consider mocking the entire http.Request.FormFile struct. However, this is an unnecessary step. The mime/multipart package offers a more efficient approach.
The mime/multipart package provides a Writer type that can generate a FormFile instance. As stated in the documentation:
CreateFormFile is a convenience wrapper around CreatePart. It creates a new form-data header with the provided field name and file name.
The CreateFormFile function returns an io.Writer that can be used to construct a FormFile field. This io.Writer can then be passed as an argument to httptest.NewRequest, which accepts a reader as an argument.
To implement this technique, one can either write the FormFile to an io.ReaderWriter buffer or utilize an io.Pipe. The following example demonstrates the latter approach:
<code class="go">import ( "fmt" "io" "io/ioutil" "net/http" "net/http/httptest" "github.com/codegangsta/multipart" ) func TestUploadFile(t *testing.T) { // Create a pipe to avoid buffering pr, pw := io.Pipe() // Create a multipart writer to transform data into multipart form data writer := multipart.NewWriter(pw) go func() { defer writer.Close() // Create the form data field 'fileupload' with a file name part, err := writer.CreateFormFile("fileupload", "someimg.png") if err != nil { t.Errorf("failed to create FormFile: %v", err) } // Generate an image dynamically and encode it to the multipart writer img := createImage() err = png.Encode(part, img) if err != nil { t.Errorf("failed to encode image: %v", err) } }() // Create an HTTP request using the multipart writer and set the Content-Type header request := httptest.NewRequest("POST", "/", pr) request.Header.Add("Content-Type", writer.FormDataContentType()) // Create a response recorder to capture the response response := httptest.NewRecorder() // Define the handler function to test handler := func(w http.ResponseWriter, r *http.Request) { // Parse the multipart form data if err := r.ParseMultipartForm(32 << 20); err != nil { http.Error(w, "failed to parse multipart form data", http.StatusBadRequest) return } // Read the uploaded file file, header, err := r.FormFile("fileupload") if err != nil { if err == http.ErrMissingFile { http.Error(w, "missing file", http.StatusBadRequest) return } http.Error(w, fmt.Sprintf("failed to read file: %v", err), http.StatusInternalServerError) return } defer file.Close() // Save the file to disk outFile, err := os.Create("./uploads/" + header.Filename) if err != nil { http.Error(w, fmt.Sprintf("failed to save file: %v", err), http.StatusInternalServerError) return } defer outFile.Close() if _, err := io.Copy(outFile, file); err != nil { http.Error(w, fmt.Sprintf("failed to copy file: %v", err), http.StatusInternalServerError) return } w.Write([]byte("ok")) } // Serve the request with the handler function handler.ServeHTTP(response, request) // Verify the response status code and file creation if response.Code != http.StatusOK { t.Errorf("incorrect HTTP status: %d", response.Code) } if _, err := os.Stat("./uploads/someimg.png"); os.IsNotExist(err) { t.Errorf("failed to create file: ./uploads/someimg.png") } else if body, err := ioutil.ReadAll(response.Body); err != nil { t.Errorf("failed to read response body: %v", err) } else if string(body) != "ok" { t.Errorf("incorrect response body: %s", body) } }</code>
This example provides a complete flow for testing an endpoint that handles file uploads, from generating a mock FormFile to asserting the response status code and file creation. By utilizing the mime/multipart package and pipes, you can efficiently simulate a request that contains uploaded files and thoroughly test your endpoints.
The above is the detailed content of How can I mock `http.Request.FormFile` in Go for testing web endpoints?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










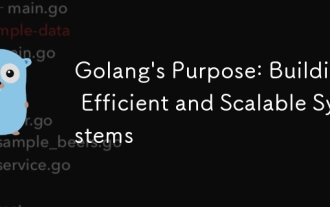
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
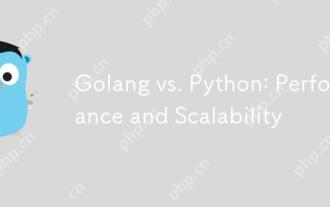
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
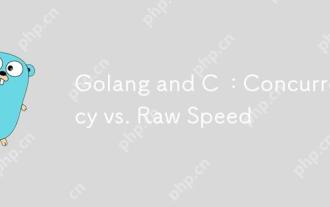
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
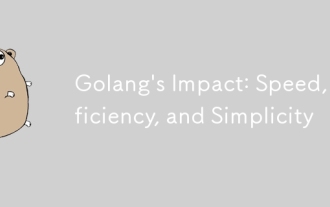
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
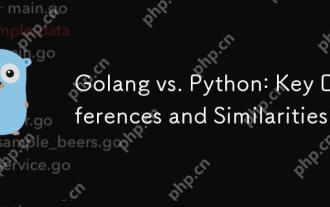
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
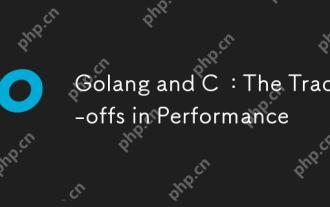
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
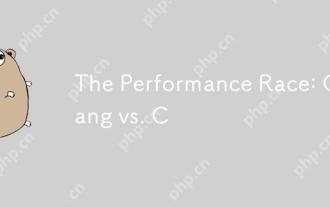
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
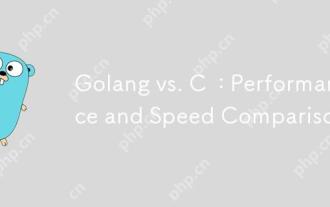
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
