How Can I Inject CSS Stylesheets as JavaScript Strings?
Injecting CSS Stylesheets as JavaScript Strings
Injecting custom CSS styles into a webpage is a crucial task when customizing the appearance of web applications. While the document.stylesheets API allows stylesheet manipulation, it presents limitations for injecting complete stylesheets as a single string. To address this challenge, we explore an alternative solution using JavaScript's powerful document manipulation capabilities.
Solution:
The recommended approach involves creating a new style element dynamically using JavaScript. By setting its textContent property with the desired CSS string, we can effectively insert a new stylesheet into the webpage's head, allowing us to apply custom styles.
<code class="javascript">// Example CSS string to add const styleString = ` body { color: red; } `; // Create a new style element and set its textContent const style = document.createElement('style'); style.textContent = styleString; // Append the style element to the head document.head.append(style);</code>
This approach provides a clean and efficient way to add custom CSS styles to a webpage, enabling developers to personalize the user interface without resorting to complex external stylesheet manipulation.
Alternative Approach:
A slightly modified version of the solution allows for replacing existing CSS styles instead of appending them. This provides more flexibility when dynamically altering the webpage's appearance.
<code class="javascript">// Example CSS string to add const styleString = ` body { background: silver; } `; // Create a utility function to handle replaceable CSS const addStyle = (() => { const style = document.createElement('style'); document.head.append(style); return (styleString) => style.textContent = styleString; })(); // Apply the style using the addStyle function addStyle(styleString);</code>
Both solutions provide effective methods for injecting CSS stylesheets as strings using JavaScript, empowering developers with the ability to customize webpage appearances dynamically.
The above is the detailed content of How Can I Inject CSS Stylesheets as JavaScript Strings?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










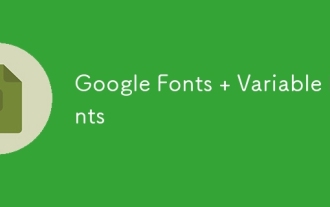
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
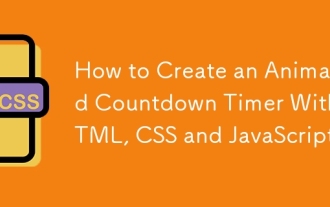
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
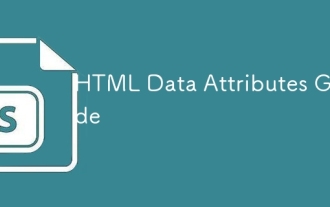
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
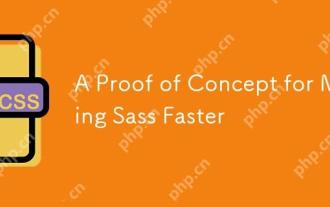
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
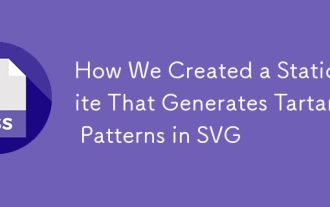
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
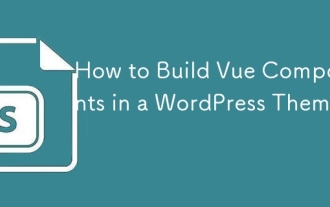
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
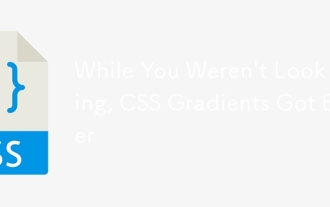
One thing that caught my eye on the list of features for Lea Verou's conic-gradient() polyfill was the last item:
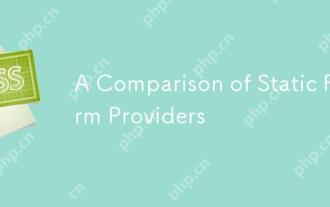
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
