


Go s Game-Changing unique Package: Supercharge Your Data Deduplication
Go 1.23 introduced a brand-new standard library package called unique, which aims to provide more efficient and lower-overhead data deduplication functionality. Here's a detailed introduction to the new features and advantages of the unique package:
Overview of the New unique Standard Library
The unique package provides a set of high-performance data deduplication tools for Go developers, applicable to various data types such as slices, strings, and more. The package achieves significant efficiency improvements and reduced runtime overhead through optimized algorithms and memory management.
Key Features
-
Multi-type Support:
- Supports deduplication of primitive data types (e.g., integers, strings).
- Supports deduplication of complex data structures (e.g., structs, slices).
-
High-performance Algorithms:
- Employs advanced hashing algorithms and parallel processing techniques to boost deduplication speed.
- Internal optimizations reduce unnecessary memory allocations and copy operations.
-
Concise and Intuitive API:
- Provides a simple function interface, making it easy to integrate into existing codebases.
- Supports chaining and functional programming styles, enhancing code readability.
-
Low Memory Overhead:
- Optimizes memory allocation and reference counting to reduce memory usage.
- Suitable for large-scale data processing scenarios, avoiding performance bottlenecks due to memory constraints.
Usage Examples
Here are some examples showcasing the usage of the unique package in different scenarios:
Example 1: Deduplicating an Integer Slice
package main import ( "fmt" "unique" ) func main() { numbers := []int{1, 2, 3, 2, 4, 3, 5} uniqueNumbers := unique.IntSlice(numbers) fmt.Println(uniqueNumbers) // Output: [1 2 3 4 5] }
Example 2: Deduplicating a String Slice
package main import ( "fmt" "unique" ) func main() { words := []string{"apple", "banana", "apple", "cherry", "banana"} uniqueWords := unique.StringSlice(words) fmt.Println(uniqueWords) // Output: ["apple", "banana", "cherry"] }
Example 3: Deduplicating a Custom Struct Slice
package main import ( "fmt" "unique" "reflect" ) type Person struct { Name string Age int } func main() { people := []Person{ {"Alice", 30}, {"Bob", 25}, {"Alice", 30}, {"Charlie", 35}, } // Use a custom equality function uniquePeople := unique.Slice(people, func(a, b Person) bool { return a.Name == b.Name && a.Age == b.Age }) fmt.Println(uniquePeople) // Output: [{Alice 30} {Bob 25} {Charlie 35}] }
Performance Comparison
Compared to the traditional method of using map for data deduplication, the unique package offers significant performance and memory usage improvements. Here's a simple performance comparison example:
Traditional map-based Deduplication
func uniqueWithMap(ints []int) []int { seen := make(map[int]struct{}) var result []int for _, num := range ints { if _, exists := seen[num]; !exists { seen[num] = struct{}{} result = append(result, num) } } return result }
unique Package Deduplication
import "unique" func uniqueWithUniquePackage(ints []int) []int { return unique.IntSlice(ints) }
For large data sets, the unique package's implementation, which optimizes the use of hash tables and memory allocation, can complete the deduplication operation faster and with lower memory usage.
Migration Guide
If you're already using custom deduplication logic or other third-party libraries in your project, you can follow these steps to migrate to the unique package:
- Upgrade to Go 1.23: Ensure that your development environment is using Go 1.23 or later.
- Update import paths: Replace your existing deduplication logic with the functions provided by the unique package.
- Test the functionality: After the migration, run tests to ensure the correctness and performance improvements of the deduplication functionality.
- Optimize the code: Based on the features of the unique package, further optimize your code structure and performance.
Conclusion
The new standard library package unique introduced in Go 1.23 provides efficient and concise data deduplication tools for developers. With its optimized algorithms and memory management, the unique package not only improves the performance of deduplication operations but also reduces memory overhead, making it suitable for various large-scale data processing scenarios. Developers are encouraged to try and integrate the unique package early on to fully leverage its performance advantages and development convenience.
The above is the detailed content of Go s Game-Changing unique Package: Supercharge Your Data Deduplication. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










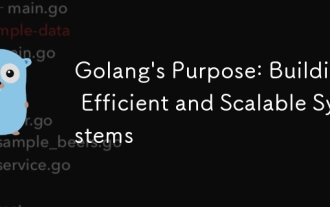
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
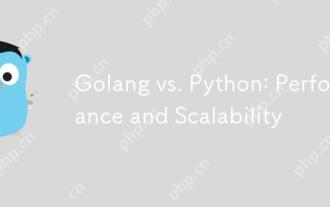
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
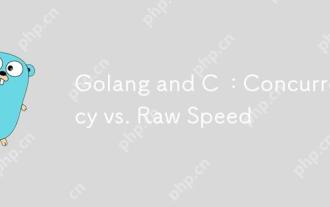
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
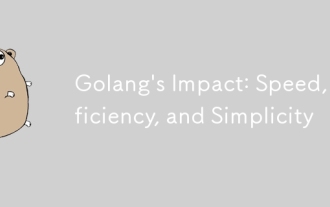
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
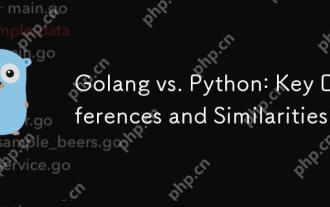
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
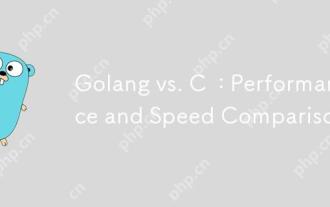
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
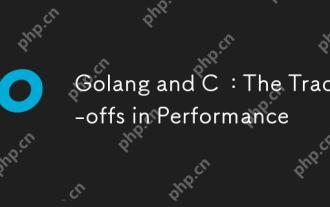
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
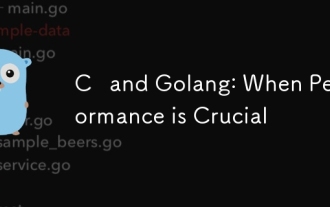
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
