How to Animate Box-Shadow with jQuery: Plugin vs. CSS Animations?
Animating Box-Shadow with jQuery: The Right Approach
Introduction
jQuery, a popular JavaScript library, provides powerful tools for manipulating DOM elements. One such manipulation is animating the box-shadow property to create visual effects. However, the correct syntax for achieving this can be confusing.
jQuery Plugin for Shadow Animation
The most straightforward method for animating box-shadow with jQuery is to utilize Edwin Martin's jQuery plugin specifically designed for this task. By extending the .animate method, it simplifies the process:
<code class="javascript">$(element).animate({ boxShadow: "0px 0px 5px 3px hsla(100, 70%, 60%, 0.8)" });</code>
Using this plugin, every aspect of the box shadow (color, offsets, radii) is animated smoothly. It also supports the animation of multiple shadows.
CSS Animations as an Alternative
While jQuery's approach is effective, it can interfere with specificity and move style information outside of stylesheets. For situations where browser support is adequate, CSS animations provide a cleaner solution:
<code class="css">@keyframes shadowPulse { ... } .shadow-pulse { ... }</code>
By applying an animation-based class with JavaScript and listening for the animationend event, you can synchronize the animation with additional actions:
Vanilla JS:
<code class="javascript">element.classList.add('shadow-pulse'); element.addEventListener('animationend', () => { // ... });</code>
jQuery:
<code class="javascript">$(element).addClass('shadow-pulse'); $(element).on('animationend', function() { // ... });</code>
The above is the detailed content of How to Animate Box-Shadow with jQuery: Plugin vs. CSS Animations?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










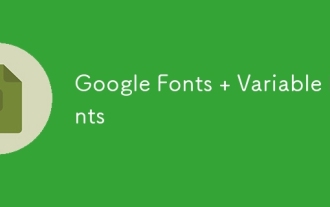
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
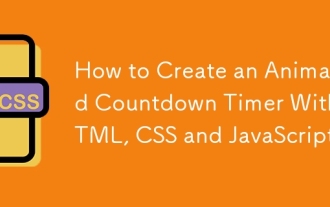
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
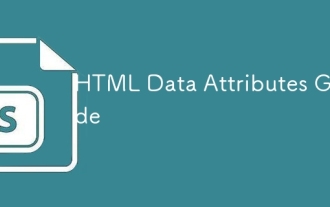
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
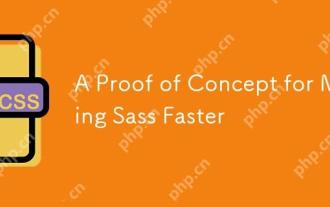
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
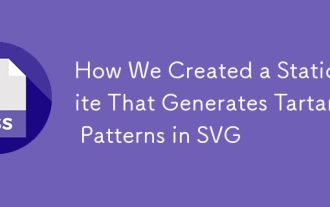
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
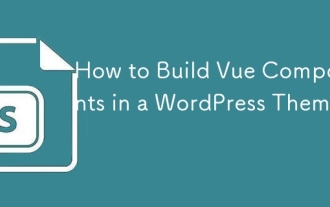
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
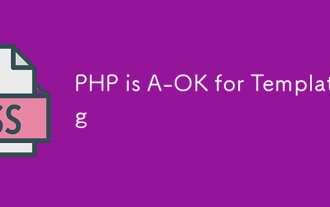
PHP templating often gets a bad rap for facilitating subpar code — but that doesn't have to be the case. Let’s look at how PHP projects can enforce a basic
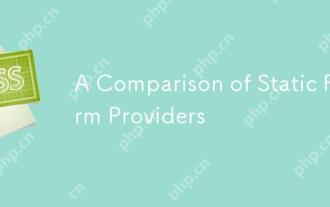
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
