How to Freeze Table Rows and Columns Using JavaScript and CSS?
How to Freeze Table Rows and Columns with JavaScript and CSS
Locking the first row and first column of a table when scrolling can be achieved using JavaScript and CSS, simulating the functionality of Excel's 'freeze panes'.
JavaScript Solution
One JavaScript solution is to create two separate tables:
- A fixed header table containing the first row and column headings.
- A scrollable body table containing the remaining table data.
Both tables are positioned absolutely using CSS, with the fixed header table positioned over the body table. When the page is scrolled, the body table moves independently while the fixed header remains fixed.
CSS Positioning
<code class="css">/* Fixed header table */ .fixed-header { position: absolute; top: 0; left: 0; z-index: 1; } /* Scrollable body table */ .scrollable-body { position: absolute; top: 0; left: 0; z-index: 0; }</code>
JavaScript Activation
<code class="javascript">// Create a table with the first row as the header const table = document.createElement('table'); const headerRow = table.createTHead().insertRow(); // Create the first column headings for (let i = 0; i < numColumns; i++) { headerRow.appendChild(document.createElement('th')).innerHTML = 'Heading ' + (i + 1); } // Create the body of the table with the remaining data const body = table.createTBody(); for (let i = 0; i < numRows; i++) { const row = body.insertRow(); for (let j = 0; j < numColumns; j++) { row.appendChild(document.createElement('td')).innerHTML = 'Data ' + (i + 1) + ', ' + (j + 1); } } // Split the table into two: fixed header and scrollable body const fixedHeader = table.cloneNode(true); fixedHeader.tBodies[0].remove(); const scrollableBody = table.cloneNode(true); fixedHeader.tHead.remove(); // Add the two tables to the page document.body.appendChild(fixedHeader); document.body.appendChild(scrollableBody); // Position the tables using CSS fixedHeader.classList.add('fixed-header'); scrollableBody.classList.add('scrollable-body');</code>
This solution provides a fully functional "freeze panes" effect for tables, ensuring that the first row and column remain visible during scrolling.
The above is the detailed content of How to Freeze Table Rows and Columns Using JavaScript and CSS?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










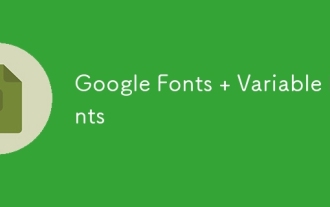
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
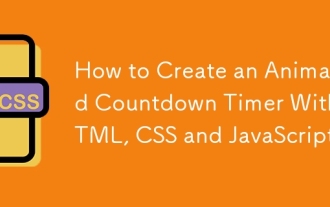
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
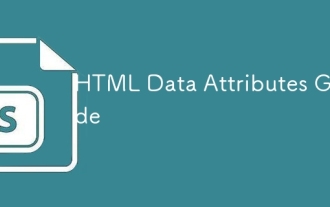
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
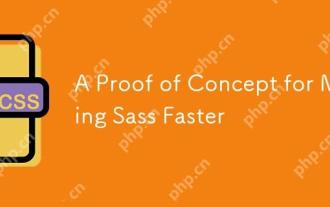
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
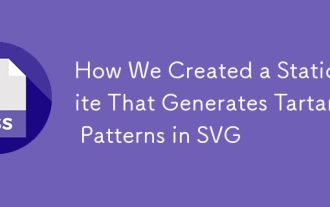
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
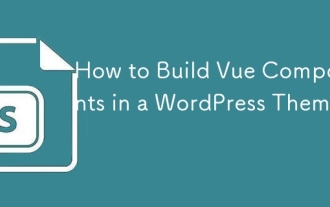
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
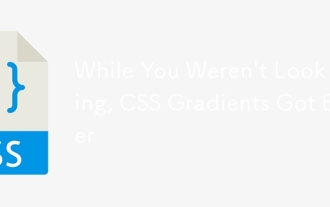
One thing that caught my eye on the list of features for Lea Verou's conic-gradient() polyfill was the last item:
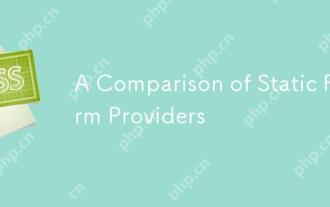
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
