


How can I effectively implement HTTP caching with PHP for dynamic websites with static content?
Integrating HTTP Caching with PHP
In situations where page content remains primarily static but surrounded by a dynamic template, leveraging HTTP cache headers can optimize page delivery. Here's a simplified guide to implementing effective caching using PHP:
Essential Headers for Caching
To enable caching, consider sending the following headers:
- Content-Type: Specify the MIME type and character set of the response.
- Vary: Accept: Indicate that the response can vary based on the client's Accept header, controlling content negotiation.
- Cache-Control: Set directives like private_no_expire to allow private caching but prevent revalidation. Alternatively, use public to allow shared caching.
- Last-Modified: Provide the date and time when the content was last modified.
- ETag: Assign a unique identifier to the content, which clients can use to determine if the cached version is still current.
Conditional Requests and Handling
Process incoming conditional requests using if-modified-since and if-none-match:
- if-modified-since: Compare the request header with the Last-Modified header. If the cached version is still valid, return a 304 (Not Modified) status code.
- if-none-match: Validate against the ETag header. If the cached version matches, return a 304.
Determining Cache Validity
When generating the ETag, consider using a checksum or a combination of factors like user ID, language, and timestamp. For longer-lasting static content, set a longer expiration.
Example Implementation
<code class="php">$tsstring = gmdate('D, d M Y H:i:s ', $timestamp) . 'GMT'; $etag = $language . $timestamp; $if_modified_since = isset($_SERVER['HTTP_IF_MODIFIED_SINCE']) ? $_SERVER['HTTP_IF_MODIFIED_SINCE'] : false; $if_none_match = isset($_SERVER['HTTP_IF_NONE_MATCH']) ? $_SERVER['HTTP_IF_NONE_MATCH'] : false; if ((($if_none_match && $if_none_match == $etag) || (!$if_none_match)) && ($if_modified_since && $if_modified_since == $tsstring)) { header('HTTP/1.1 304 Not Modified'); exit(); } else { header("Last-Modified: $tsstring"); header("ETag: \"{$etag}\""); }</code>
The above is the detailed content of How can I effectively implement HTTP caching with PHP for dynamic websites with static content?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


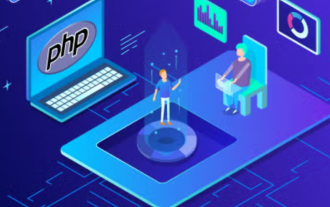
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
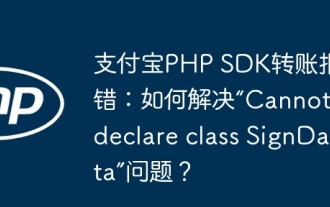
Alipay PHP...
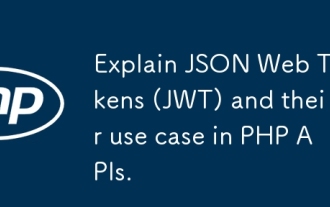
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
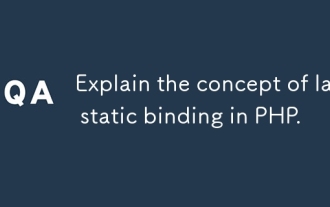
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
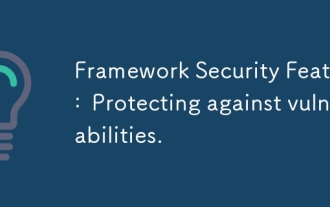
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
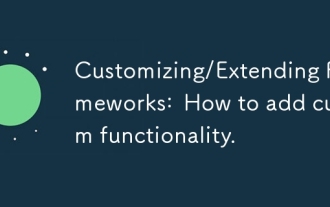
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
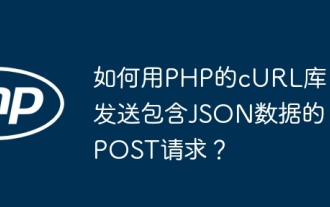
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
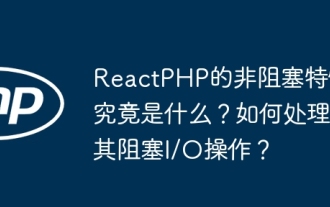
An official introduction to the non-blocking feature of ReactPHP in-depth interpretation of ReactPHP's non-blocking feature has aroused many developers' questions: "ReactPHPisnon-blockingbydefault...
