How to Decrypt AES Data in ECB Mode Using Go?
Decrypting AES Data in ECB Mode Using Go
In response to the query about decrypting data using AES-ECB in Go, the following solution can be employed:
Electronic codebook (ECB) mode is a fundamental加密方法 that divides data into blocks of a specified size (e.g., AES-128 uses 16-byte blocks). Each block is then encrypted independently using the AES algorithm, resulting in the encrypted block.
To decrypt data encrypted using AES-128 ECB, follow these steps:
-
Import necessary module:
<code class="go">import ( "crypto/aes" )</code>
Copy after login -
Create a new cipher:
<code class="go">cipher, _ := aes.NewCipher([]byte(key))</code>
Copy after loginReplace key with the encryption key used to encrypt the data.
-
Create an empty buffer to store the decrypted data:
<code class="go">decrypted := make([]byte, len(data))</code>
Copy after logindata represents the encrypted data.
-
Define the block size:
<code class="go">size := 16 // block size for AES-128</code>
Copy after login -
Decrypt each block:
<code class="go">for bs, be := 0, size; bs < len(data); bs, be = bs+size, be+size { cipher.Decrypt(decrypted[bs:be], data[bs:be]) }</code>
Copy after loginThis loop decrypts each block and stores the result in the decrypted buffer.
-
Return the decrypted data:
<code class="go">return decrypted</code>
Copy after login
Remember that ECB mode has known security vulnerabilities, as identical blocks will always result in the same encrypted blocks. Consider other modes of operation, such as CBC or GCM, for more secure encryption with AES.
The above is the detailed content of How to Decrypt AES Data in ECB Mode Using Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
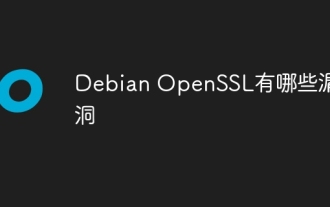
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
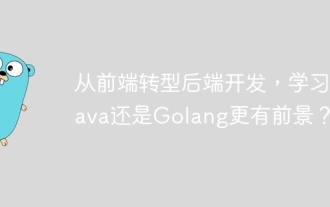
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
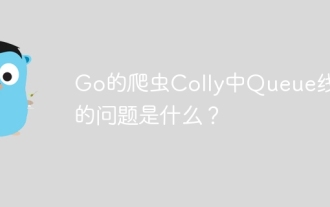
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
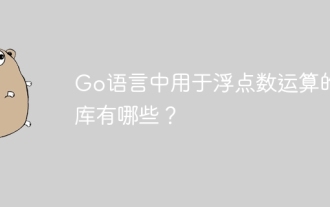
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
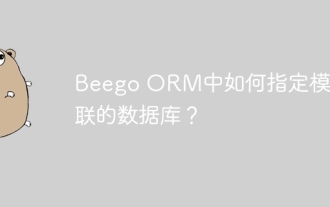
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
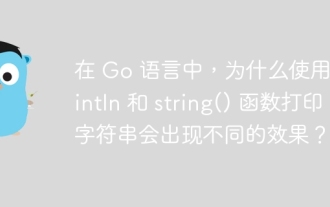
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
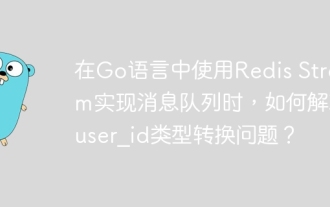
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
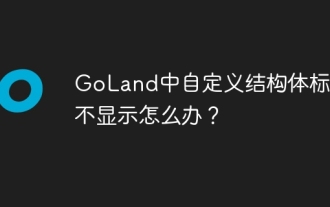
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
