How do you create a simple SSH port forward in Golang?
Simple SSH Port Forward in Golang
In this article, we will explore how to create a simple SSH port forward, allowing you to connect to a remote port from your local machine.
Creating a Port Forward
To create a port forward, you need to establish an SSH connection and listen on the local port. Once a connection is made, you can forward data between the local and remote ports.
Here's a step-by-step guide:
- Set up SSH configuration: Create a ssh.ClientConfig object with the necessary authentication information (username, password, server address).
- Listen on the local port: Create a net.Listener object to listen for incoming connections on the desired local port.
- Accept incoming connections: Use the listener.Accept() method to accept incoming local connections.
- Connect to the remote server: Use the provided ssh.ClientConfig object to establish an SSH connection to the remote server.
- Connect to the remote port: Establish a connection to the specified remote port on the server.
- Forward data: Use io.Copy() to continuously forward data from the local connection to the remote connection and vice versa.
Example Code
The following Go code demonstrates these steps:
<code class="go">package main import ( "io" "log" "net" "golang.org/x/crypto/ssh" ) func forward(localConn net.Conn, config *ssh.ClientConfig, remoteAddr string) { sshClientConn, err := ssh.Dial("tcp", "remote.example.com:22", config) if err != nil { log.Fatalf("ssh.Dial failed: %s", err) } sshConn, err := sshClientConn.Dial("tcp", remoteAddr) if err != nil { log.Fatalf("sshClientConn.Dial failed: %s", err) } go io.Copy(sshConn, localConn) go io.Copy(localConn, sshConn) } func main() { config := &ssh.ClientConfig{ User: "username", Auth: []ssh.AuthMethod{ ssh.Password("password"), }, } listener, err := net.Listen("tcp", "local.example.com:9000") if err != nil { log.Fatalf("net.Listen failed: %s", err) } for { localConn, err := listener.Accept() if err != nil { log.Fatalf("listener.Accept failed: %s", err) } go forward(localConn, config, "remote.example.com:9999") } }</code>
By following these steps and using the provided code, you can create a simple yet effective SSH port forward and access remote services from your local machine with ease.
The above is the detailed content of How do you create a simple SSH port forward in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










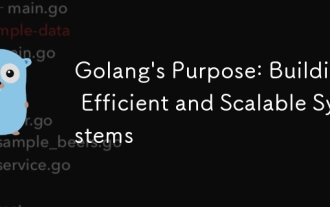
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
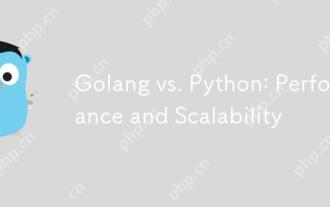
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
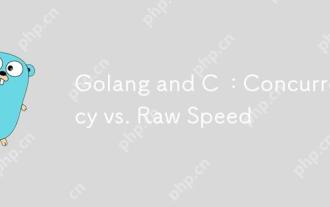
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
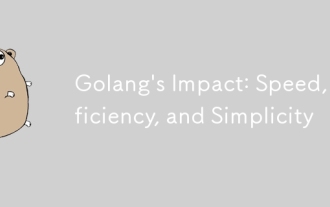
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
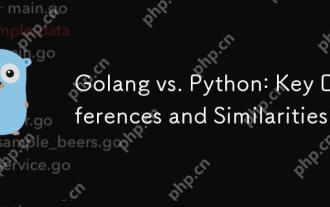
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
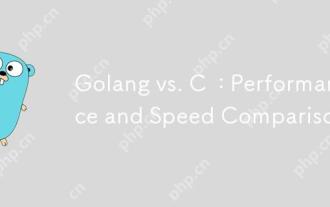
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
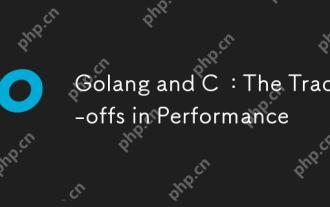
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
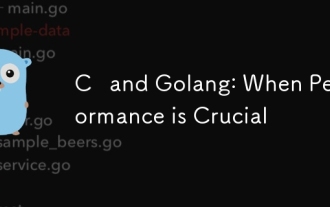
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
