


How can we efficiently split a text string of concatenated words without spaces into individual words?
Splitting Text into a Word List Without Spaces
Problem
Given a text string consisting of concatenated words without spaces:
Input: "tableapplechairtablecupboard..."
How can we efficiently split this text into a list of individual words?
Output: ["table", "apple", "chair", "table", ["cupboard", ["cup", "board"]], ...]
Algorithm
A simple approach is to iteratively find the longest possible word within the text. However, this can lead to suboptimal results.
Frequency-Based Algorithm
Instead, we can exploit the relative frequency of words in the language to improve accuracy:
- Model the Word Distribution: Assume words are independently distributed and follow Zipf's law, where word probability is inversely proportional to its rank.
- Define Word Cost: The cost of a word is defined as the logarithm of the inverse of its likelihood.
-
Dynamic Programming Approach:
- Initialize a cost array where the first element is 0.
- For each character in the text, find the word that minimizes the total cost for characters up to that point.
- Backtrack from the end to reconstruct the minimum-cost word sequence.
Code Implementation
<code class="python">from math import log wordcost = {} # Dictionary of word costs using Zipf's law maxword = max(len(word) for word in wordcost) def infer_spaces(s): cost = [0] for i in range(1, len(s) + 1): candidates = enumerate(reversed(cost[max(0, i - maxword):i])) c, k = min((wordcost.get(s[i - k - 1:i], 9e999) + c, k + 1) for k, c in candidates) cost.append(c) out = [] i = len(s) while i > 0: c, k = best_match(i) assert c == cost[i] out.append(s[i - k:i]) i -= k return " ".join(reversed(out))</code>
Results
This algorithm is able to accurately segment text into a list of words, even in the absence of spaces.
Example:
Input: "tableapplechairtablecupboard..." Output: ["table", "apple", "chair", "table", ["cupboard", ["cup", "board"]], ...]
Optimizations:
- Suffix Tree: By building a suffix tree from the word list, the candidate search can be accelerated.
- Text Block Splitting: For large text inputs, the text can be split into blocks to minimize memory usage while maintaining accuracy.
The above is the detailed content of How can we efficiently split a text string of concatenated words without spaces into individual words?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










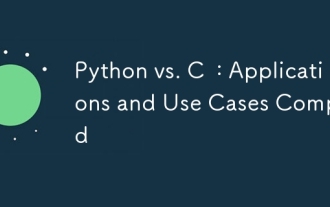
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
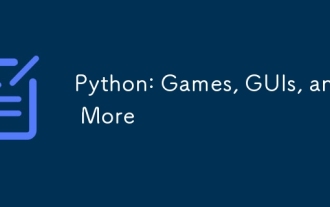
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
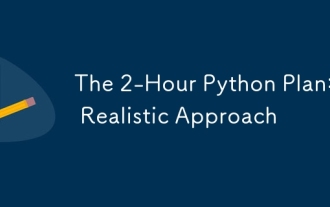
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
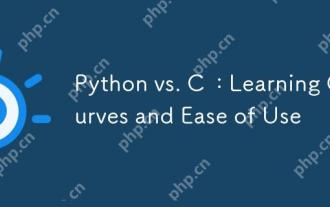
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
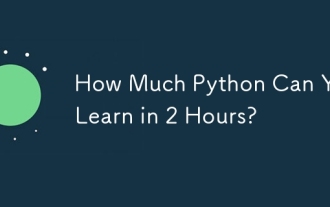
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
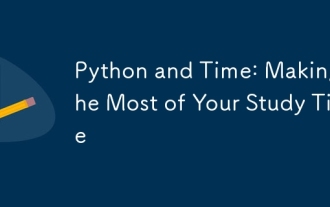
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
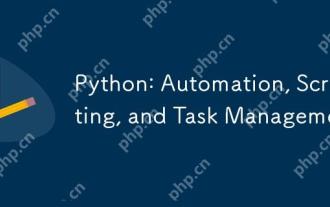
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
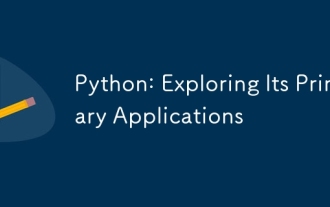
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
