


How to Add a Custom Attribute to a Laravel/Eloquent Model on Load?
Nov 04, 2024 pm 01:15 PMAdd a Custom Attribute to a Laravel/Eloquent Model on Load
Problem Analysis
In Laravel/Eloquent, custom attributes not directly related to a column in the underlying table are excluded when converting models to toArray() or JSON format.
Solution
Laravel 8 and above:
Use the Attribute class to create a custom accessor that automatically calculates and assigns the attribute when the object loads.
<code class="php">class EventSession extends Eloquent { public function availability() { return new Attribute( get: fn () => $this->calculateAvailability() ); } }</code>
Laravel 7 and below:
Option 1: Append Attribute to $appends Property
Add the custom attribute to the $appends array and define an appropriate accessor.
<code class="php">class EventSession extends Eloquent { protected $appends = ['availability']; public function getAvailabilityAttribute() { return $this->calculateAvailability(); } }</code>
Option 2: Override toArray() Method
Override the toArray() method and explicitly set or iterate over the custom attributes.
<code class="php">class Book extends Eloquent { public function toArray() { $array = parent::toArray(); $array['upper'] = $this->upper; return $array; } public function getUpperAttribute() { return strtoupper($this->title); } }</code>
Option 3: Loop Through Mutated Attributes
Loop through the model's mutated attributes and apply them to the toArray() array.
<code class="php">class Book extends Eloquent { public function toArray() { $array = parent::toArray(); foreach ($this->getMutatedAttributes() as $key) { if (!array_key_exists($key, $array)) { $array[$key] = $this->{$key}; } } return $array; } public function getUpperAttribute() { return strtoupper($this->title); } }</code>
The above is the detailed content of How to Add a Custom Attribute to a Laravel/Eloquent Model on Load?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
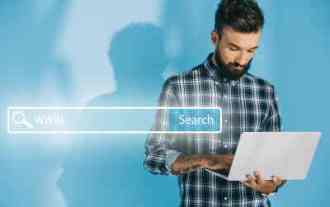
11 Best PHP URL Shortener Scripts (Free and Premium)
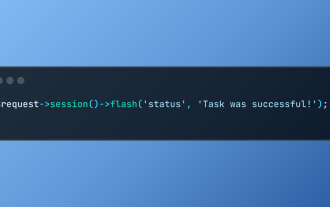
Working with Flash Session Data in Laravel
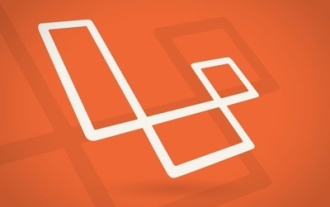
Build a React App With a Laravel Back End: Part 2, React
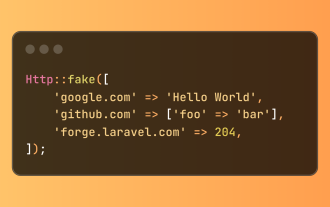
Simplified HTTP Response Mocking in Laravel Tests
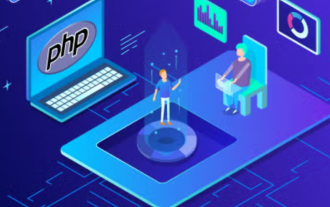
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
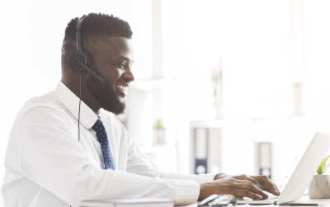
12 Best PHP Chat Scripts on CodeCanyon
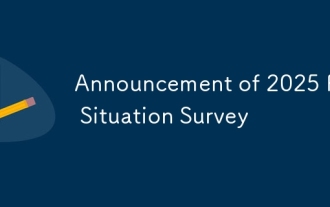
Announcement of 2025 PHP Situation Survey
