


How to Fix 'Assignment to Entry in Nil Map' Error When Creating a Slice of Maps in Go?
Runtime Error: "Assignment to Entry in Nil Map" Resolved
When attempting to create a slice of Maps, you may encounter the runtime error "assignment to entry in nil map." This error indicates that you are trying to access a nil Map value, which is not permitted.
Problem Statement
You are experiencing this error while building an array of Maps, each containing two keys: "Id" and "Investor." The attempted code is as follows:
<code class="go">for _, row := range rows { invs := make([]map[string]string, length) for i := 0; i < length; i++ { invs[i] = make(map[string]string) invs[i]["Id"] = inv_ids[i] invs[i]["Investor"] = inv_names[i] } }</code>
Resolution
To resolve this error, you should create a slice of Maps directly within the loop instead of creating nil Maps and assigning values to them. This can be achieved using composite literals:
<code class="go">for _, row := range rows { invs := make([]map[string]string, length) for i := 0; i < length; i++ { invs[i] = map[string]string{"Id": inv_ids[i], "Investor": inv_names[i]} } }</code>
Alternative Approach
Alternatively, you can use a struct to represent an investor:
<code class="go">type Investor struct { Id int Investor string } for _, row := range rows { invs := make([]Investor, length) for i := 0; i < length; i++ { invs[i] = Investor{ Id: inv_ids[i], Investor: inv_names[i], } } }</code>
Using a struct provides a cleaner and more structured representation of your data.
The above is the detailed content of How to Fix 'Assignment to Entry in Nil Map' Error When Creating a Slice of Maps in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










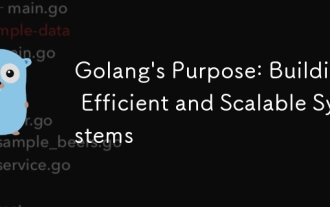
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
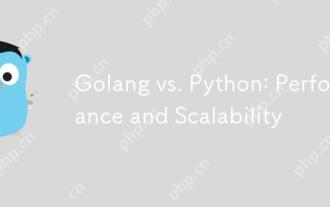
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
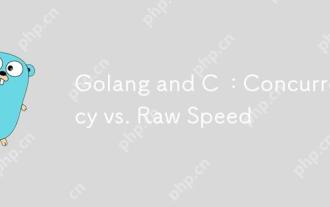
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
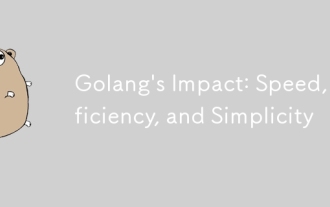
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
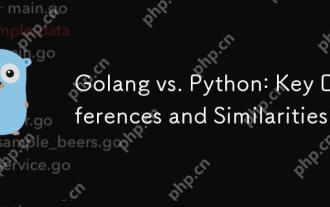
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
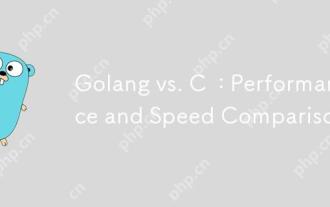
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
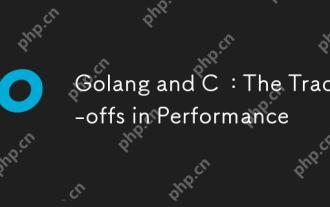
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
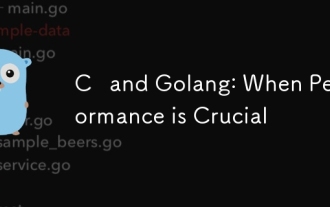
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
