How to Efficiently Map Prime Numbers within a Range?
Efficiently Mapping Prime Numbers within a Range
Determining prime numbers within a specified range is a common programming task. To optimize memory consumption for this task, we seek an algorithm that creates the most compact data structure that represents prime numbers for a given range (1, N].
Proposed Algorithm for Prime Range Mapping
The most effective algorithm for general prime testing is the AKS algorithm. However, for practical purposes within a limited range, the following variant of the classic O(sqrt(N)) algorithm can provide an efficient solution:
def isprime(n): if n == 2 or n == 3: return True if n % 2 == 0 or n % 3 == 0: return False # Check prime divisors of the form 6k - 1 and 6k + 1 i = 5 w = 2 while i * i <= n: if n % i == 0: return False i += w w = 6 - w return True
Analysis of the Algorithm
This algorithm relies on the fact that all prime numbers greater than 3 are either of the form 6k - 1 or 6k 1. By iterating through potential prime divisors in this pattern, the algorithm efficiently identifies non-prime numbers.
Additional Considerations
For even more speed, especially when the range is limited, implementing a pseudo-prime test based on Fermat's little theorem can be effective. However, this approach has a range limitation.
Key Optimization
The most significant optimization in this algorithm is the elimination of all even numbers as potential primes. This optimization significantly reduces the number of checks required, leading to improved performance.
The above is the detailed content of How to Efficiently Map Prime Numbers within a Range?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










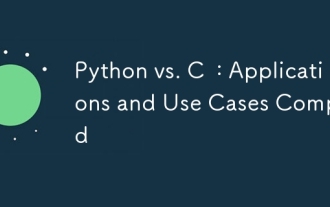
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
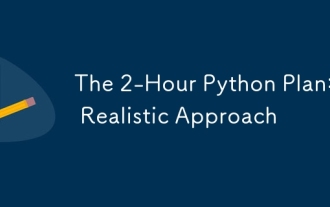
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
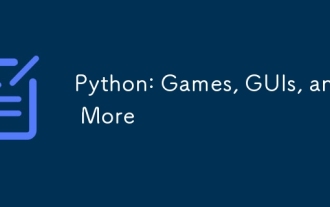
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
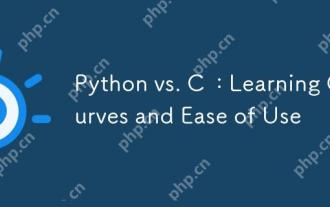
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
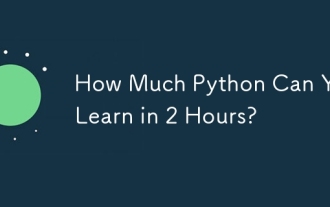
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
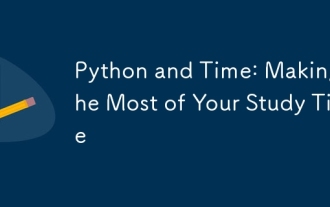
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
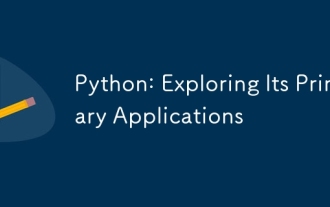
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
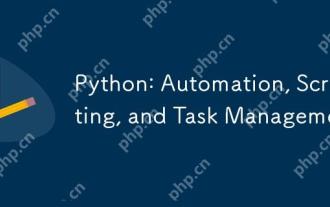
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
